C#: Check whether a given string contain the character 'z' from 2 to 4 times
C# Sharp Basic Algorithm: Exercise-22 with Solution
Write a C# Sharp program to check if a given string contains between 2 and 4 'z' characters.
Visual Presentation:
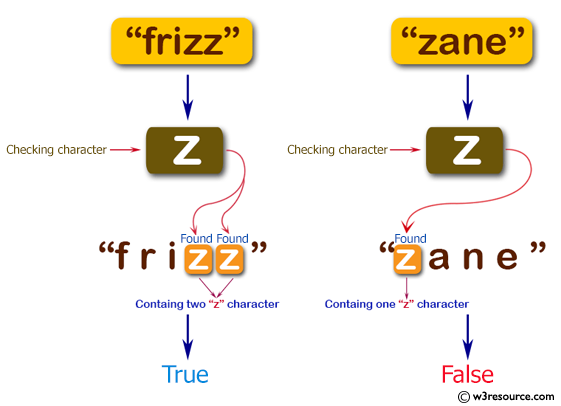
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different strings
Console.WriteLine(test("frizz")); // Output: True
Console.WriteLine(test("zane")); // Output: True
Console.WriteLine(test("Zazz")); // Output: False
Console.WriteLine(test("false")); // Output: False
Console.WriteLine(test("zzzz")); // Output: True
Console.WriteLine(test("ZZZZ")); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to check if the input string contains 'z' from 2 to 4 times
public static bool test(string str)
{
int ctr = 0; // Counter to store the number of occurrences of 'z'
// Loop through each character in the input string
for (int i = 0; i < str.Length; i++)
{
// Check if the current character is 'z'
if (str[i] == 'z')
{
ctr++; // Increment the counter if the character is 'z'
}
}
// Return true if the count of 'z' is greater than 1 and less than or equal to 4, otherwise return false
return ctr > 1 && ctr <= 4;
}
}
}
Sample Output:
True False True False True False
Flowchart:
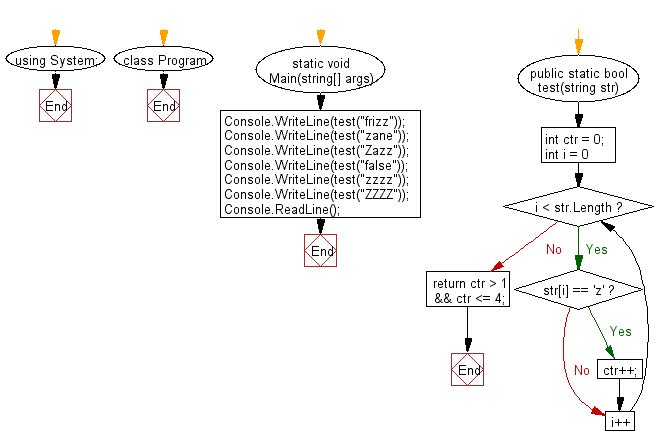
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to find the larger value from two positive integer values that is in the range 20..30 inclusive, or return 0 if neither is in that range.
Next: Write a C# Sharp program to check if two given non-negative integers have the same last digit
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics