C#: Check the largest number among three given integers
C# Sharp Basic Algorithm: Exercise-18 with Solution
Write a C# Sharp program to check the largest number among three given integers.
Visual Presentation:
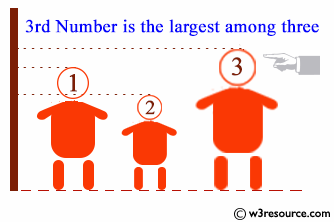
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different integer values
Console.WriteLine(test(1, 2, 3)); // Output: 3
Console.WriteLine(test(1, 3, 2)); // Output: 3
Console.WriteLine(test(1, 1, 1)); // Output: 1
Console.WriteLine(test(1, 2, 2)); // Output: 2
Console.ReadLine(); // Keeping the console window open
}
// Method to find the maximum value among three integers
public static int test(int x, int y, int z)
{
// Using Math.Max method to find the maximum value between x, y, and z
// Math.Max is used twice to find the maximum value among the three integers
var max = Math.Max(x, Math.Max(y, z));
// Returning the maximum value among the three integers
return max;
}
}
}
Sample Output:
3 3 1 2
Flowchart:
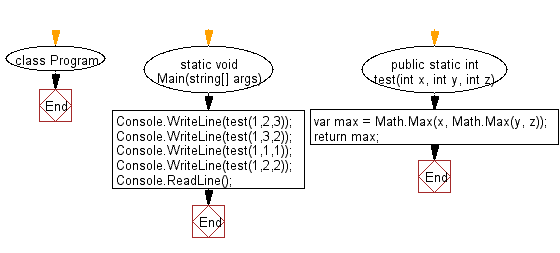
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check if a string 'yt' appears at index 1 in a given string. If it appears return a string without 'yt' otherwise return the original string.
Next: Write a C# Sharp program to check which number nearest to the value 100 among two given integers. Return 0 if the two numbers are equal.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics