C#: Check whether a string 'yt' appears at index 1 in a given string
Remove 'yt' at Index 1
Write a C# Sharp program to check whether a string 'yt' appears at index 1 in a given string. If it appears return a string without 'yt' otherwise return the original string.
Visual Presentation:
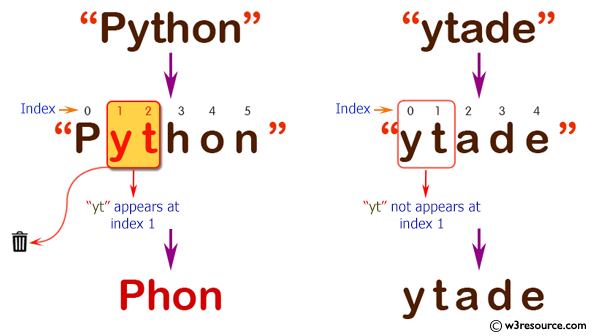
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different strings
Console.WriteLine(test("Python")); // Output: Pyhon
Console.WriteLine(test("ytade")); // Output: yade
Console.WriteLine(test("jsues")); // Output: jsues
Console.ReadLine(); // Keeping the console window open
}
// Method to perform a specific operation on the input string
public static string test(string str)
{
// Check if substring from index 1 to index 2 (exclusive) is "yt"
// If true, remove characters at index 1 and 2 from the string; otherwise, return the original string
return str.Substring(1, 2).Equals("yt") ? str.Remove(1, 2) : str;
}
}
}
Sample Output:
Phon ytade jsues
Flowchart:
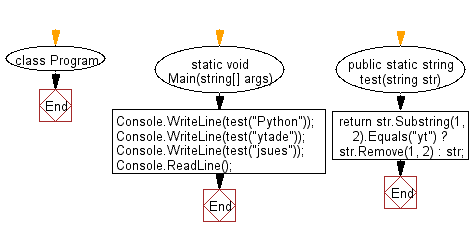
For more Practice: Solve these Related Problems:
- Write a C# program to remove the substring 'at' if it appears at index 1 in a given string.
- Write a C# program to remove a specific 2-character substring starting at index 2 if it matches 'pp'.
- Write a C# program to remove all occurrences of 'yt' starting at index 1 or 2.
- Write a C# program to remove the substring 'yt' only if it is surrounded by vowels in the original string.
Go to:
PREV : One of Two Integers in Range 20-50.
NEXT : Largest Among Three Integers.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.