C#: Check whether three given integer values are in the range 20..50 inclusive
Check Integers in Range 20-50
Write a C# Sharp program to check whether three given integer values are in the range 20..50 inclusive. Return true if 1 or more of them are in the said range otherwise false.
Visual Presentation:
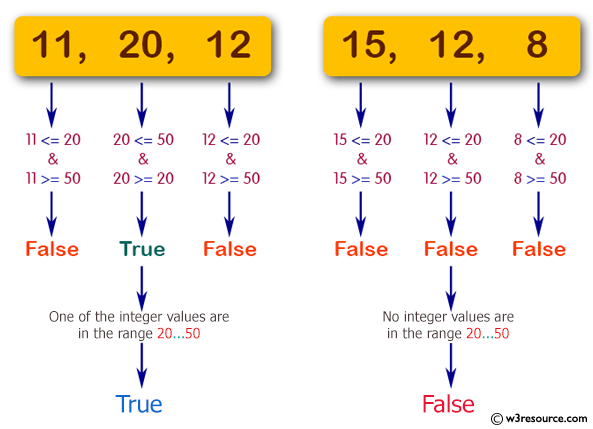
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the results of the 'test' method with different integer trios
Console.WriteLine(test(11, 20, 12)); // Output: True
Console.WriteLine(test(30, 30, 17)); // Output: True
Console.WriteLine(test(25, 35, 50)); // Output: True
Console.WriteLine(test(15, 12, 8)); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to test if at least one number in a trio is within the range 20 to 50 (inclusive)
public static bool test(int x, int y, int z)
{
// Check if x is in the range [20, 50] OR y is in the range [20, 50] OR z is in the range [20, 50]
// Return true if at least one of x, y, or z (or more) is within the specified range; otherwise, return false
return (x >= 20 && x <= 50) || (y >= 20 && y <= 50) || (z >= 20 && z <= 50);
}
}
}
Sample Output:
True True True False
Flowchart:
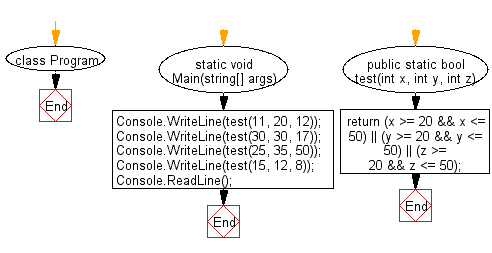
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check two given integers whether either of them is in the range 100..200 inclusive.
Next: Write a C# Sharp program to check whether two given integer values are in the range 20..50 inclusive. Return true if 1 or other is in the said range otherwise false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.