C#: Check two given integers whether either of them is in the range 100..200 inclusive
C# Sharp Basic Algorithm: Exercise-14 with Solution
Write a C# Sharp program to check two given integers whether either of them is in the range 100..200 inclusive.
Visual Presentation:
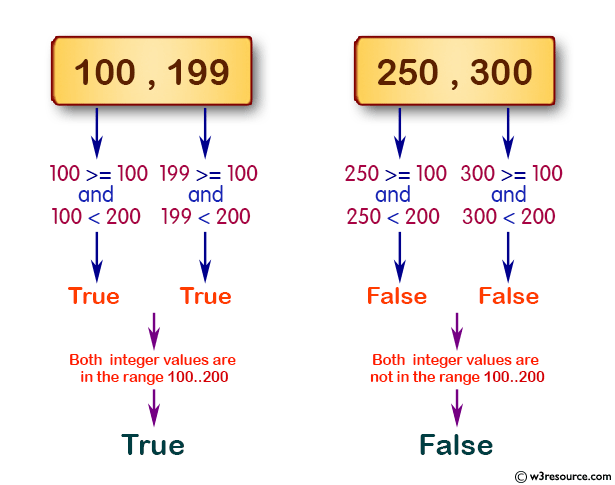
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the results of the 'test' method with different integer pairs
Console.WriteLine(test(100, 199)); // Output: True
Console.WriteLine(test(250, 300)); // Output: False
Console.WriteLine(test(105, 190)); // Output: True
Console.ReadLine(); // Keeping the console window open
}
// Method to test if at least one number is within the range 100 to 200 (inclusive)
public static bool test(int x, int y)
{
// Check if x is in the range [100, 200] OR y is in the range [100, 200]
// Return true if either x or y (or both) are within the specified range; otherwise, return false
return (x >= 100 && x <= 200) || (y >= 100 && y <= 200);
}
}
}
Sample Output:
True False True
Flowchart:
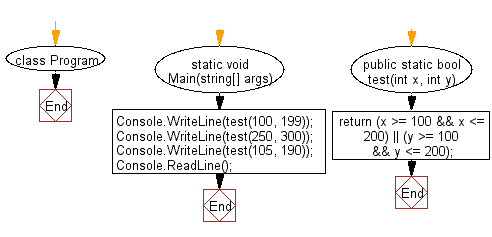
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check if one given temperatures is less than 0 and the other is greater than 100.
Next: Write a C# Sharp program to check whether three given integer values are in the range 20..50 inclusive. Return true if 1 or more of them are in the said range otherwise false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-14.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics