C#: Check a given array of integers and return true if the given array contains either 2 even or 2 odd values all next to each other
Two Consecutive Even or Odd Values
Write a C# Sharp program to check a given array of integers. Return true if the given array contains 2 even or 2 odd values all next to each other.
Visual Presentation:
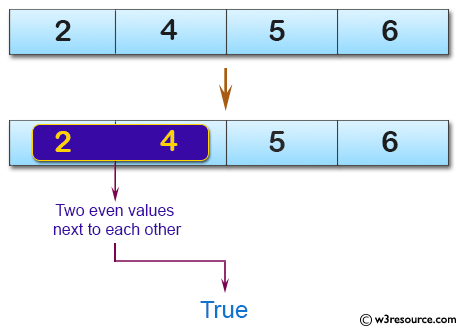
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 3, 5, 1, 3, 7 })); // Testing the method with an array that satisfies the condition
Console.WriteLine(test(new[] { 1, 2, 3, 4 })); // Testing the method with an array that doesn't satisfy the condition
Console.WriteLine(test(new[] { 3, 3, 5, 5, 5, 5 })); // Testing the method with an array that satisfies the condition with multiple occurrences
Console.WriteLine(test(new[] { 2, 4, 5, 6 })); // Testing the method with an array that doesn't satisfy the condition
}
// Method to check if an array contains consecutive occurrences of two odd or two even numbers
static bool test(int[] numbers)
{
int tot_odd = 0, tot_even = 0; // Variables to count the consecutive odd and even numbers encountered
for (int i = 0; i < numbers.Length; i++) // Looping through the elements of the array
{
if (tot_odd < 2 && tot_even < 2) // Checking if the total count of consecutive odd and even numbers is less than 2
{
if (numbers[i] % 2 == 0) // If the number is even
{
tot_even++; // Increment the count of consecutive even numbers
tot_odd = 0; // Reset the count of consecutive odd numbers
}
else // If the number is odd
{
tot_odd++; // Increment the count of consecutive odd numbers
tot_even = 0; // Reset the count of consecutive even numbers
}
}
}
// Return true if there are two consecutive odds or two consecutive evens, otherwise false
return tot_odd == 2 || tot_even == 2;
}
}
}
Sample Output:
True False True True
Flowchart:
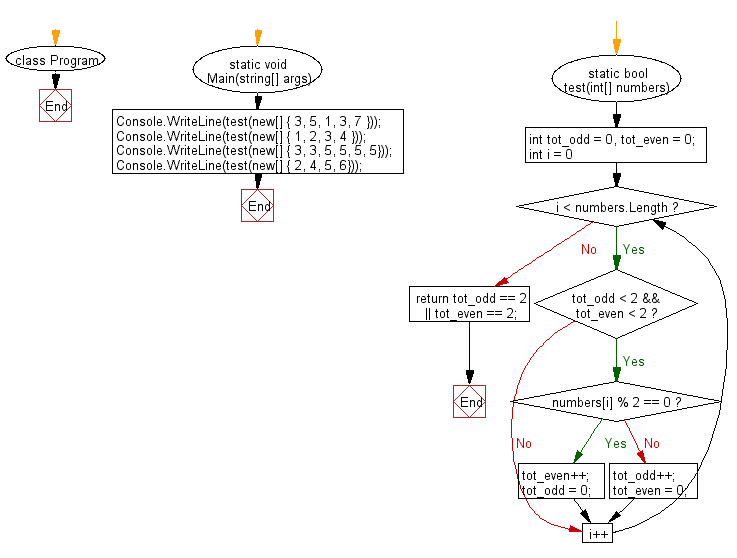
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check a given array of integers and return true if there is a 3 with a 5 somewhere later in the given array.
Next: Check a given array of integers and return true if the value 5 appears 5 times and there are no 5 next to each other.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.