C#: Check a given array of integers and return true if there is a 3 with a 5 somewhere later in the given array
Check for 3 Before 5 in Array
Write a C# Sharp program to check a given array of integers and return true if there is a 3 with a 5 somewhere later in the given array.
Visual Presentation:
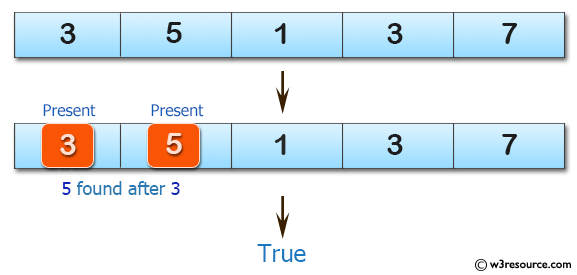
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 3, 5, 1, 3, 7 })); // Testing the method with an array having 3 followed by 5
Console.WriteLine(test(new[] { 1, 2, 3, 4 })); // Testing the method with an array without 3 followed by 5
Console.WriteLine(test(new[] { 3, 3, 5, 5, 5, 5 })); // Testing the method with an array having multiple 3s and 5s
Console.WriteLine(test(new[] { 2, 5, 5, 7, 8, 10 })); // Testing the method with an array having 5s but no 3 followed by 5
}
// Method to check if an array contains a 5 following a 3
static bool test(int[] numbers)
{
bool three = false; // Variable to track if a 3 is encountered
for (int i = 0; i < numbers.Length; i++) // Loop through the elements of the array
{
if (three && numbers[i] == 5) return true; // If a 5 is encountered after a 3, return true
if (numbers[i] == 3) three = true; // If a 3 is encountered, set the 'three' flag to true
}
return false; // If no 5 follows a 3, return false
}
}
}
Sample Output:
True False True False
Flowchart:
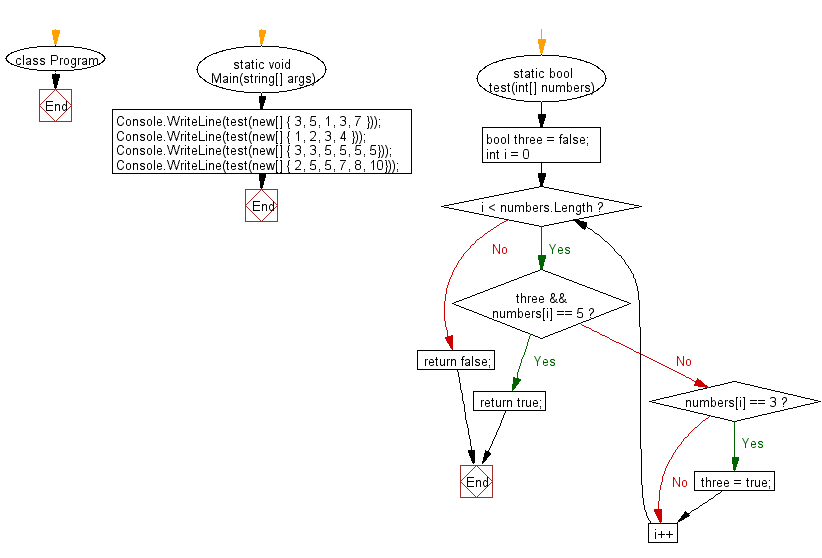
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check a given array of integers and return true if the given array contains two 5's next to each other, or two 5 separated by one element.
Next: Write a C# Sharp program to check a given array of integers and return true if the given array contains either 2 even or 2 odd values all next to each other.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.