C#: Compute the sum of the two given arrays of integers, length 3 and find the array which has the largest sum
Largest Sum Between Two Arrays
Write a C# Sharp program to compute the sum of the two given arrays of integers, length 3. Find the array with the largest sum.
Visual Presentation:
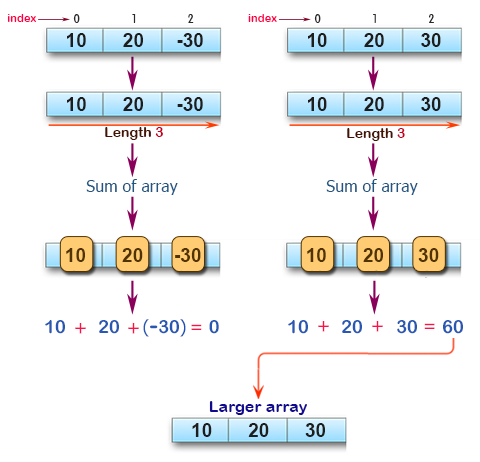
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with two integer arrays as arguments and assigning the result to the 'item' array
int[] item = test(new[] { 10, 20, -30 }, new[] { 10, 20, 30 });
// Outputting text to indicate the start of displaying the larger array
Console.Write("Larger array: ");
// Looping through each element of the 'item' array and displaying them
foreach(var i in item)
{
Console.Write(i.ToString()+" "); // Displaying each element of the 'item' array separated by a space
}
}
// Method to compare the sum of elements in two arrays and return the array with the larger sum
public static int[] test(int[] nums1, int[] nums2)
{
// Checking if the sum of elements in 'nums1' is greater than or equal to the sum of elements in 'nums2'
// If true, return 'nums1'; otherwise, return 'nums2'
return nums1[0] + nums1[1] + nums1[2] >= nums2[0] + nums2[1] + nums2[2] ? nums1 : nums2;
}
}
}
Sample Output:
Larger array: 10 20 30
Flowchart:
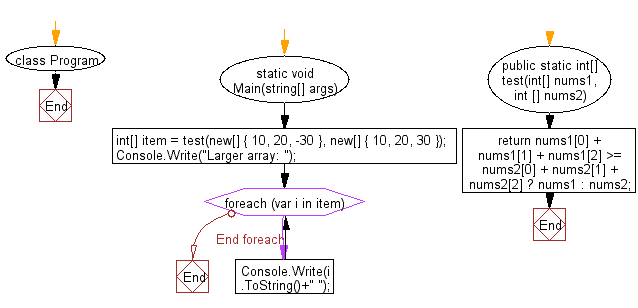
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check a given array of integers, length 3 and create a new array. If there is a 5 in the given array immediately followed by a 7 then set 7 to 1.
Next: Write a C# Sharp program to create an array taking two middle elements from a given array of integers of length even.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.