Swift String Exercises: Create a new string made of 2 copies of the first 2 characters of a given string
Write a Swift program to create a new string made of 2 copies of the first 2 characters of a given string. The string may be any length.
Pictorial Presentation:
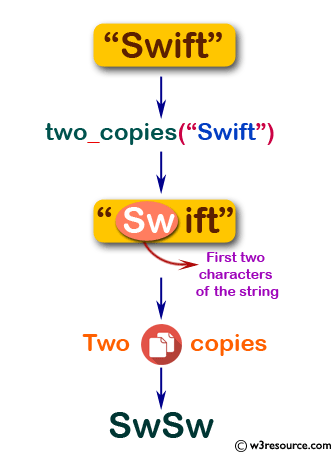
Sample Solution:
Swift Code:
import Foundation
func two_copies(_ str1: String) -> String {
if str1.characters.count == 0 {
return ""
}
else if str1.characters.count == 1
{
return str1 + str1
}
else
{
var currentIndex = str1.index(after: str1.startIndex)
currentIndex = str1.index(after: currentIndex)
let res = str1.substring(to: currentIndex)
return res + res
}
}
print(two_copies("Swift"))
print(two_copies("Online"))
print(two_copies("JS"))
Sample Output:
SwSw OnOn JSJS
Go to:
PREV : Write a Swift program to create a new string of any length from a given string where the last two characters are swapped, so 'abcde' will be 'abced'.
NEXT : Write a Swift program to check if the first or last characters are 'a' of a given string, return the given string without those 'a' characters, and otherwise return the given string.
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?