Swift String Exercises: Test if a given string starts with 'ab'
Write a Swift program to test if a given string starts with "ab".
Pictorial Presentation:
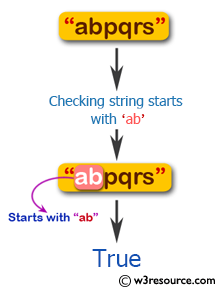
Sample Solution:
Swift Code:
import Foundation
func starts_with_ab(_ input: String) -> Bool {
if input.hasPrefix("ab")
{
return true
}
else
{
return false
}
}
print(starts_with_ab("pqrsab"))
print(starts_with_ab("abpqrsab"))
print(starts_with_ab("pqrs"))
print(starts_with_ab("abpqrs"))
Sample Output:
false true false true
Go to:
PREV : Write a Swift program to create a new string without the first and last character of a given string. The string may be any length, including 0.
NEXT : Write a Swift program to create a new string made of the first and last n chars from a given string. The string length will be at least n.
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?