Swift String Exercises: Create a new string taking the middle two characters of a given string of even length
Write a Swift program to create a new string taking the middle two characters of a given string of even length. The given string length must be at least 2.
Pictorial Presentation:
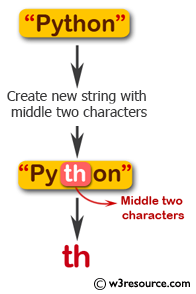
Sample Solution:
Swift Code:
import Foundation
func middleTwo(_ str: String) -> String {
var end_index = str.endIndex
var start_index = str.startIndex
while end_index != start_index {
start_index = str.index(after: start_index)
end_index = str.index(before: end_index)
}
end_index = str.index(after: end_index)
start_index = str.index(before: start_index)
return str.substring(with: Range(uncheckedBounds: (start_index, end_index)))
}
print(middleTwo("Python"))
print(middleTwo("Java"))
Sample Output:
th av
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Swift program to create a new string without the first and last character of a given string. The string may be any length, including 0.
Next: Write a Swift program to test if a given string starts with "ab".
What is the difficulty level of this exercise?