Swift Basic Programming Exercise: Check if the first instance of "a" in a given string is immediately followed by another "a"
Write a Swift program to check if the first instance of "a" in a given string is immediately followed by another "a".
Pictorial Presentation:
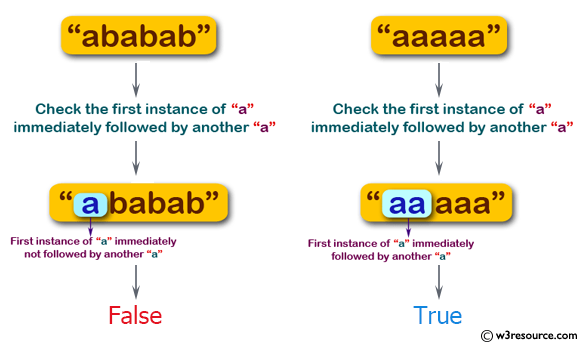
Sample Solution:
Swift Code:
func double_a(_ input: String) -> Bool {
let chars = input.characters
var ans: Bool = false
for i in chars {
let found_Index = chars.index(of: i)
let next_Index = chars.index(after: found_Index!)
if i == "a" && chars[next_Index] == "a"
{
ans = true
}
}
return ans
}
print(double_a("abbcaad"))
print(double_a("ababab"))
print(double_a("aaaaa"))
Sample Output:
false false true
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Swift program to convert the last three characters in upper case.
Next: Write a Swift program to create a string made of every other char starting with the first from a given string.
What is the difficulty level of this exercise?