Swift Basic Programming Exercise: Find the largest number among three given integers
Write a Swift program to find the largest number among three given integers.
Pictorial Presentation:
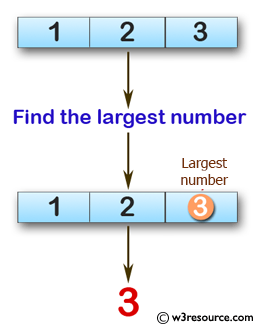
Sample Solution:
Swift Code:
func max_three(_ x: Int, _ y: Int, _ z: Int) -> Int {
if x > y, x > z
{
return x
}
else if y > z, y > x
{
return y
}
else if z > y, z > x
{
return z
}
else if x == y, y > z
{
return x
}
else if y == z, z > x
{
return y
}
else
{
return x
}
}
print(max_three(1, 2, 3))
print(max_three(3, 2, 1))
print(max_three(-3, -2, 0))
Sample Output:
3 3 0
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Swift program to check if a given string begins with "fix", except the 'f' can be any character or number.
Next: Write a Swift program that accept two integer values and to test which value is nearest to the value 10, or return 0 if both integers have same distance from 10.
What is the difficulty level of this exercise?