Swift Array Programming Exercises: Swap the first and last elements of a given array of integers
Write a Swift program to swap the first and last elements of a given array of integers. Return the modified array (length will be at least 1).
Pictorial Presentation:
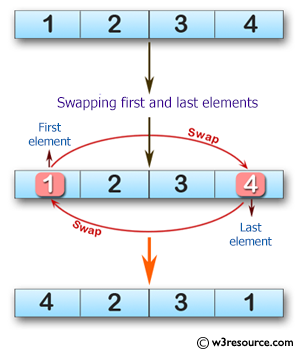
Sample Solution:
Swift Code:
func swap_elements(_ a: [Int]) -> [Int] {
var new_array = a
let first_element = new_array.removeFirst()
let last_element = new_array.removeLast()
new_array.insert(last_element, at: new_array.startIndex)
new_array.append(first_element)
return new_array
}
print(swap_elements([1, 2, 3, 4]))
print(swap_elements([1, 2, 3]))
print(swap_elements([11, 12, 13, 14, 15]))
Sample Output:
[4, 2, 3, 1] [3, 2, 1] [15, 12, 13, 14, 11]
Go to:
PREV : Write a Swift program to test if an array of length four containing all their elements from two given array (each length two) of integers,.
NEXT : Write a Swift program to create a new array of length 3 containing the elements from the middle of a given array of integers and length will be at least 3.
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?