SQL Exercises: Find salesmen who earned the maximum commission.
SQL SUBQUERY : Exercise-9 with Solution
9. From the following tables, write a SQL query to find those salespeople who earned the maximum commission. Return ord_no, purch_amt, ord_date, and salesman_id.
Sample table: Salesmansalesman_id name city commission ----------- ---------- ---------- ---------- 5001 James Hoog New York 0.15 5002 Nail Knite Paris 0.13 5005 Pit Alex London 0.11 5006 Mc Lyon Paris 0.14 5003 Lauson Hen San Jose 0.12 5007 Paul Adam Rome 0.13Sample table: Orders
ord_no purch_amt ord_date customer_id salesman_id ---------- ---------- ---------- ----------- ----------- 70001 150.5 2012-10-05 3005 5002 70009 270.65 2012-09-10 3001 5005 70002 65.26 2012-10-05 3002 5001 70004 110.5 2012-08-17 3009 5003 70007 948.5 2012-09-10 3005 5002 70005 2400.6 2012-07-27 3007 5001 70008 5760 2012-09-10 3002 5001 70010 1983.43 2012-10-10 3004 5006 70003 2480.4 2012-10-10 3009 5003 70012 250.45 2012-06-27 3008 5002 70011 75.29 2012-08-17 3003 5007 70013 3045.6 2012-04-25 3002 5001
Sample Solution:
-- Selecting specific columns from the 'orders' table
SELECT ord_no, purch_amt, ord_date, salesman_id
-- Specifying the table to retrieve data from ('orders')
FROM orders
-- Filtering the results based on the condition that 'salesman_id' is in the result of a subquery
WHERE salesman_id IN (
-- Subquery: Selecting 'salesman_id' from the 'salesman' table where 'commission' is equal to the maximum 'commission' in the 'salesman' table
SELECT salesman_id
FROM salesman
WHERE commission = (
-- Subquery: Selecting the maximum 'commission' from the 'salesman' table
SELECT MAX(commission)
FROM salesman
)
);
Output of the Query:
ord_no | purch_amt | ord_date | salesman_id --------+-----------+------------+------------- 70002 | 65.26 | 2012-10-05 | 5001 70005 | 2400.60 | 2012-07-27 | 5001 70008 | 5760.00 | 2012-09-10 | 5001 70013 | 3045.60 | 2012-04-25 | 5001 (4 rows)
Explanation:
The said SQL statement is selecting certain columns (ord_no, purch_amt, ord_date, salesman_id) from a table called 'orders', and only returning the rows where the "salesman_id" matches the "salesman_id" of a salesman with the highest commission in another table called 'salesman'.
Visual Explanation:
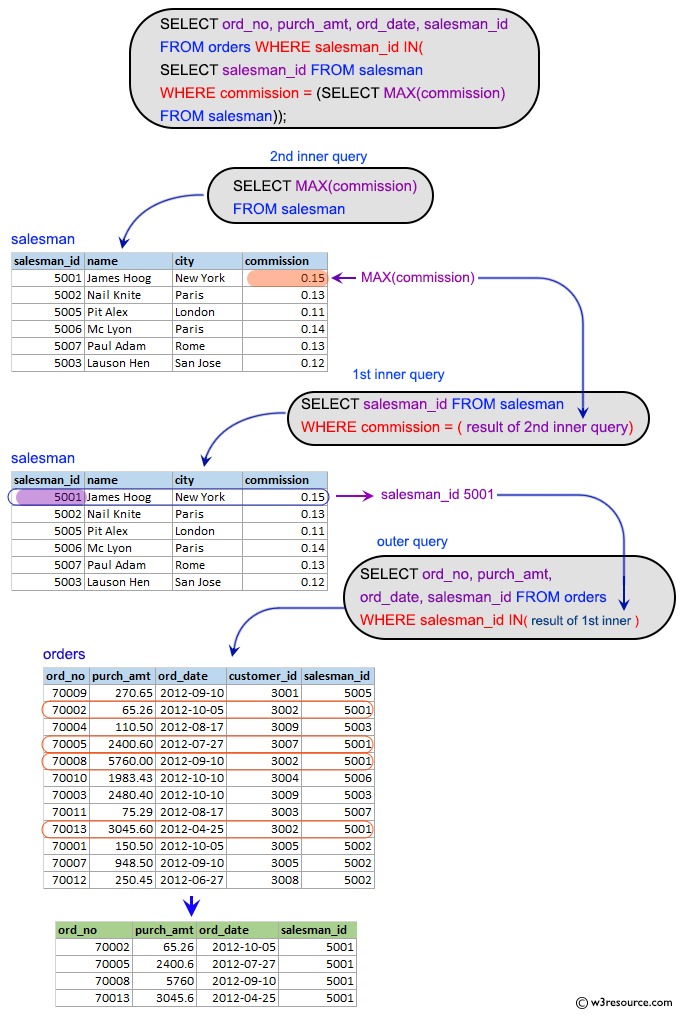
Practice Online
Sample Database: inventory
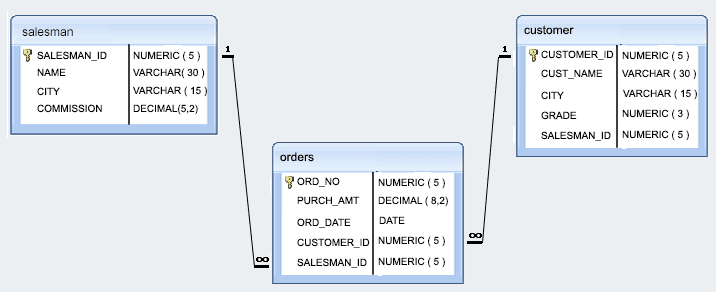
Contribute your code and comments through Disqus.
Previous SQL Exercise: Counts the customers with grades over New York average.
Next SQL Exercise: Customers who ordered on August 17, 2012.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/sql-exercises/subqueries/sql-subqueries-inventory-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics