SQL Exercises: Find all orders with above-average amounts
12. From the following tables write a SQL query to find those orders, which are higher than the average amount of the orders. Return ord_no, purch_amt, ord_date, customer_id and salesman_id.
Sample table: Ordersord_no purch_amt ord_date customer_id salesman_id ---------- ---------- ---------- ----------- ----------- 70001 150.5 2012-10-05 3005 5002 70009 270.65 2012-09-10 3001 5005 70002 65.26 2012-10-05 3002 5001 70004 110.5 2012-08-17 3009 5003 70007 948.5 2012-09-10 3005 5002 70005 2400.6 2012-07-27 3007 5001 70008 5760 2012-09-10 3002 5001 70010 1983.43 2012-10-10 3004 5006 70003 2480.4 2012-10-10 3009 5003 70012 250.45 2012-06-27 3008 5002 70011 75.29 2012-08-17 3003 5007 70013 3045.6 2012-04-25 3002 5001
Sample Solution:
-- Selecting all columns from the 'orders' table (aliased as 'a')
SELECT *
-- Specifying the table to retrieve data from ('orders' as 'a')
FROM orders a
-- Filtering the results based on the condition that 'purch_amt' is greater than the average 'purch_amt' for the same 'customer_id'
WHERE purch_amt >
-- Subquery: Calculating the average 'purch_amt' from the 'orders' table (aliased as 'b') for the same 'customer_id'
(SELECT AVG(purch_amt) FROM orders b
WHERE b.customer_id = a.customer_id);
Output of the Query:
ord_no purch_amt ord_date customer_id salesman_id 70008 5760.00 2012-09-10 3002 5001 70003 2480.40 2012-10-10 3009 5003 70013 3045.60 2012-04-25 3002 5001 70007 948.50 2012-09-10 3005 5002
Explanation:
The said SQL query is selecting all columns from the 'orders' table where the value in the "purch_amt" column is greater than the average "purch_amt" of orders associated with the same customer.
It is using a subquery in the WHERE clause to calculate the average "purch_amt" of orders for each customer by matching the "customer_id" in the 'orders' table with the "customer_id" in the 'orders' table in the subquery. The outer query then selects all columns from the 'orders' table where the value in the "purch_amt" column is greater than the average "purch_amt" returned by the subquery for that specific customer.
This results in a list of all orders with a purchase amount greater than the average purchase amount of all orders for that specific customer.
Visual Explanation:
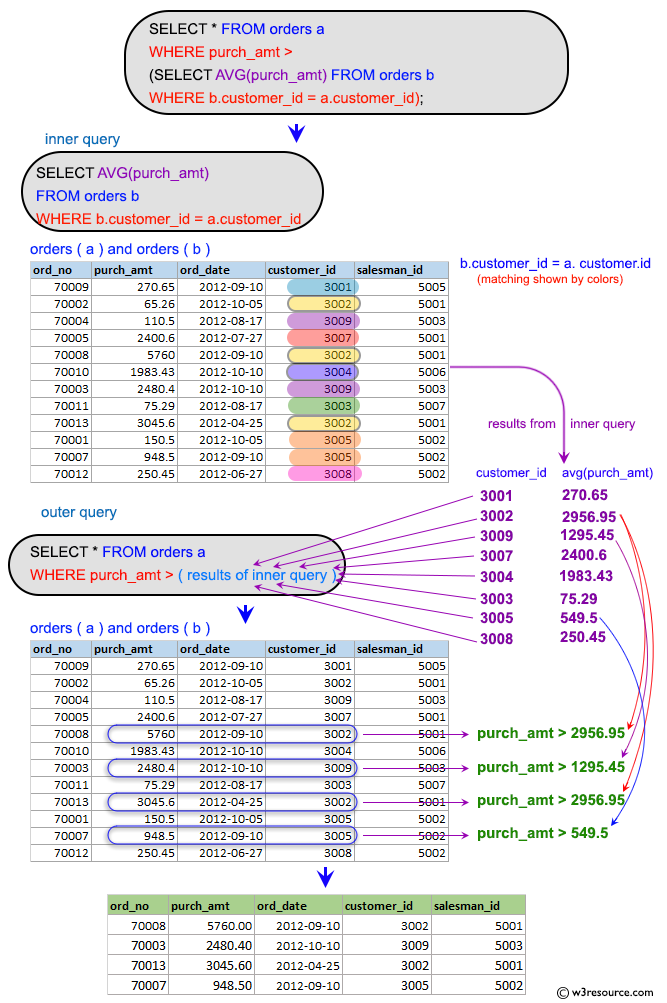
Practice Online
Sample Database: inventory
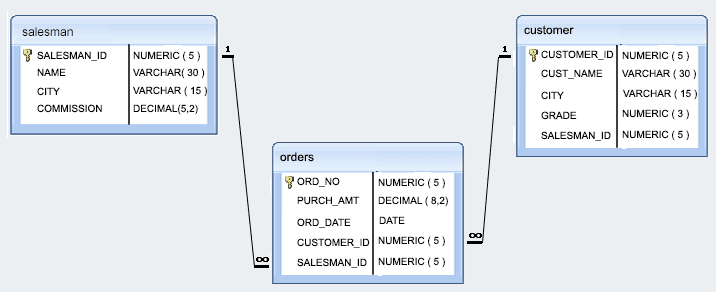
Query Visualization:
Duration:
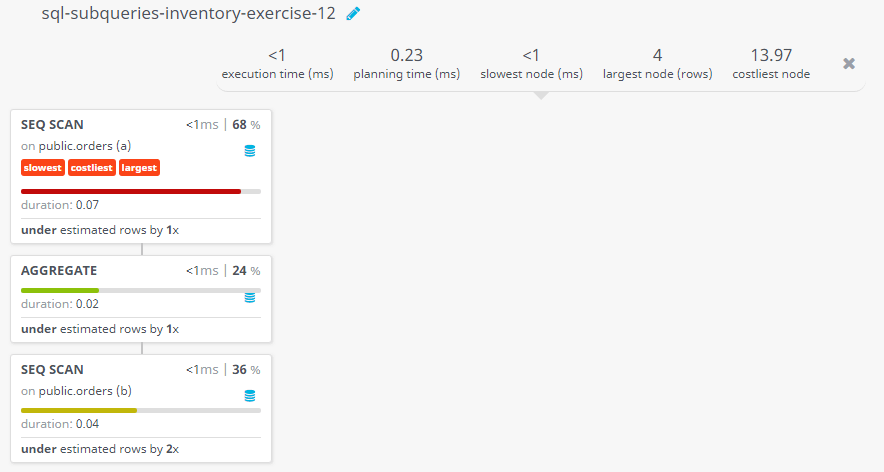
Rows:
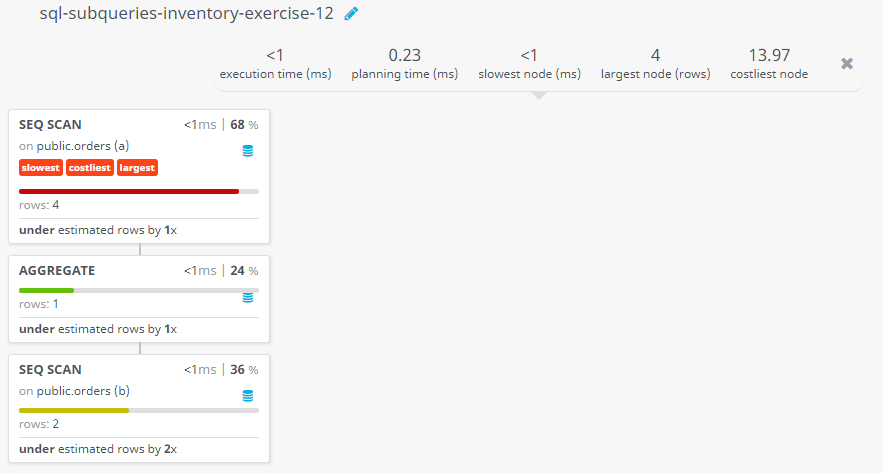
Cost:
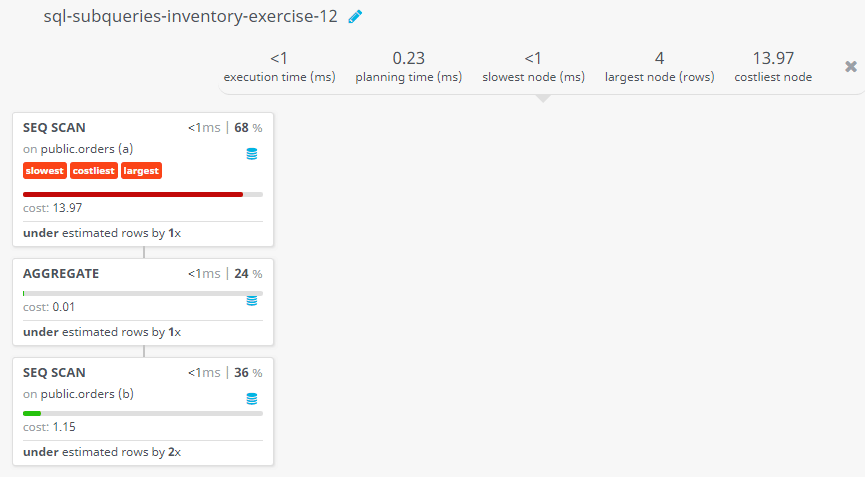
Contribute your code and comments through Disqus.
Previous SQL Exercise: List all salesmen with more than one customer.
Next SQL Exercise: Customer order amounts that are on or above average.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics