SQL Exercises: Find salesman commission details of given customer
From the following tables, write a SQL query to find all orders executed by the salesperson and ordered by the customer whose grade is greater than or equal to 200. Compute purch_amt*commission as “Commission”. Return customer name, commission as “Commission%” and Commission.
Sample table: salesman
salesman_id | name | city | commission -------------+------------+----------+------------ 5001 | James Hoog | New York | 0.15 5002 | Nail Knite | Paris | 0.13 5005 | Pit Alex | London | 0.11 5006 | Mc Lyon | Paris | 0.14 5007 | Paul Adam | Rome | 0.13 5003 | Lauson Hen | San Jose | 0.12
Sample table: customer
customer_id | cust_name | city | grade | salesman_id -------------+----------------+------------+-------+------------- 3002 | Nick Rimando | New York | 100 | 5001 3007 | Brad Davis | New York | 200 | 5001 3005 | Graham Zusi | California | 200 | 5002 3008 | Julian Green | London | 300 | 5002 3004 | Fabian Johnson | Paris | 300 | 5006 3009 | Geoff Cameron | Berlin | 100 | 5003 3003 | Jozy Altidor | Moscow | 200 | 5007 3001 | Brad Guzan | London | | 5005
Sample table: orders
ord_no purch_amt ord_date customer_id salesman_id ---------- ---------- ---------- ----------- ----------- 70001 150.5 2012-10-05 3005 5002 70009 270.65 2012-09-10 3001 5005 70002 65.26 2012-10-05 3002 5001 70004 110.5 2012-08-17 3009 5003 70007 948.5 2012-09-10 3005 5002 70005 2400.6 2012-07-27 3007 5001 70008 5760 2012-09-10 3002 5001 70010 1983.43 2012-10-10 3004 5006 70003 2480.4 2012-10-10 3009 5003 70012 250.45 2012-06-27 3008 5002 70011 75.29 2012-08-17 3003 5007 70013 3045.6 2012-04-25 3002 5001
Sample Solution:
-- This query selects specific columns ('ord_no', 'cust_name', 'commission' with alias "Commission%",
-- and 'purch_amt * commission' with alias "Commission") from the 'salesman', 'orders', and 'customer' tables.
-- It retrieves data where the 'customer_id' column in the 'orders' table matches the 'customer_id' column in the 'customer' table,
-- the 'salesman_id' column in the 'orders' table matches the 'salesman_id' column in the 'salesman' table,
-- and the 'grade' column in the 'customer' table is greater than or equal to 200.
SELECT ord_no, cust_name, commission AS "Commission%", purch_amt * commission AS "Commission"
-- Specifies the tables from which to retrieve the data (in this case, 'salesman', 'orders', and 'customer').
FROM salesman, orders, customer
-- Specifies the conditions for joining the tables and filtering the data.
WHERE orders.customer_id = customer.customer_id
AND orders.salesman_id = salesman.salesman_id
AND customer.grade >= 200;
Output of the query:
ord_no cust_name Commission% Commission 70005 Brad Davis 0.15 360.0900 70010 Fabian Johnson 0.14 277.6802 70011 Jozy Altidor 0.13 9.7877 70001 Graham Zusi 0.13 19.5650 70007 Graham Zusi 0.13 123.3050 70012 Julian Green 0.13 32.5585
Code Explanation:
The said query in SQL that retrieves the order number, customer name, commission rate as "Commission%", and the calculated commission amount for salesmen who have customers with a grade of 200 or higher.
By joining the salesman, order, and customer tables on their respective ID fields, the query returns the columns that are specified in the SELECT statement for the salesman, order, and customer tables.
The WHERE clause filters the result set to only include orders made by customers with a grade of 200 or higher.
Explanation:
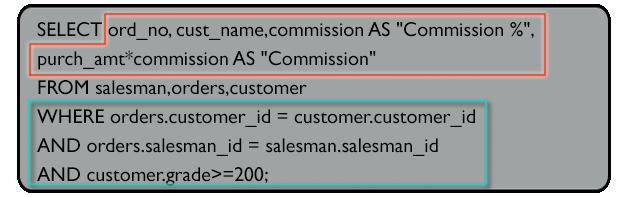
Visual presentation:
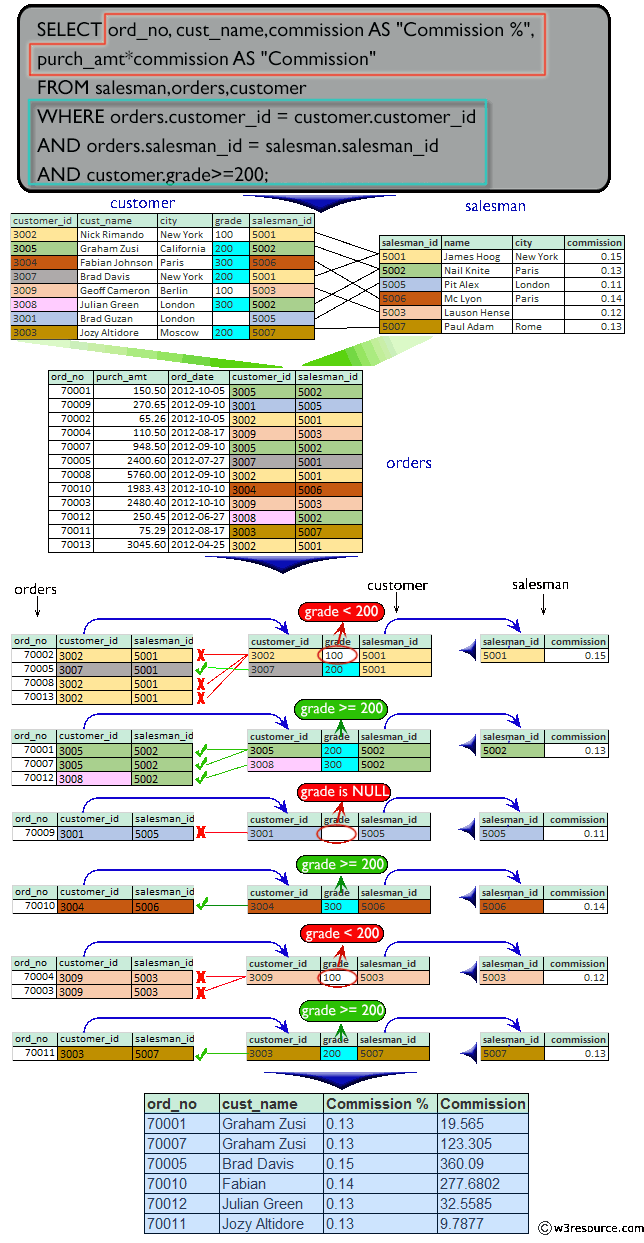
Practice Online
Query Visualization:
Duration:
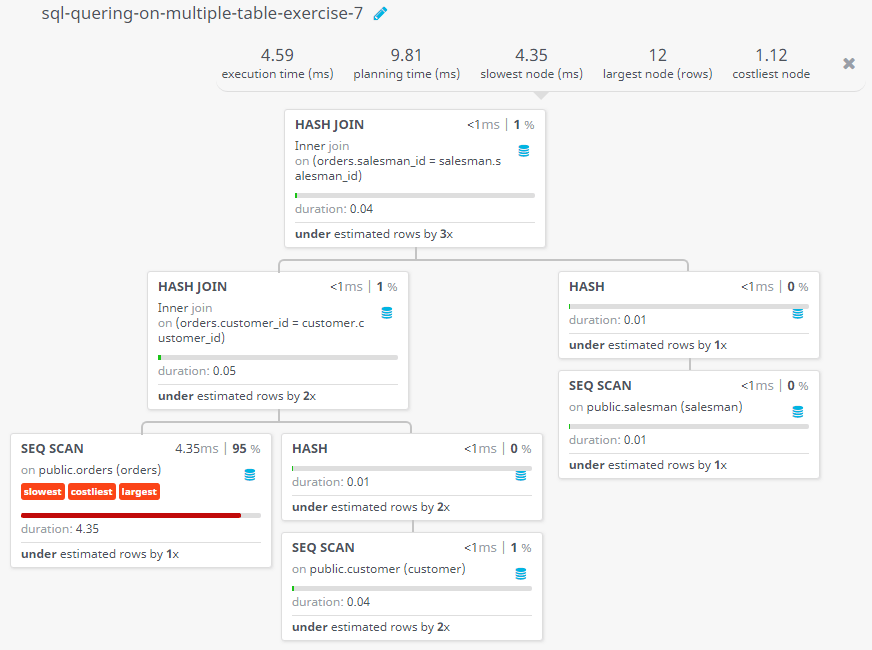
Rows:
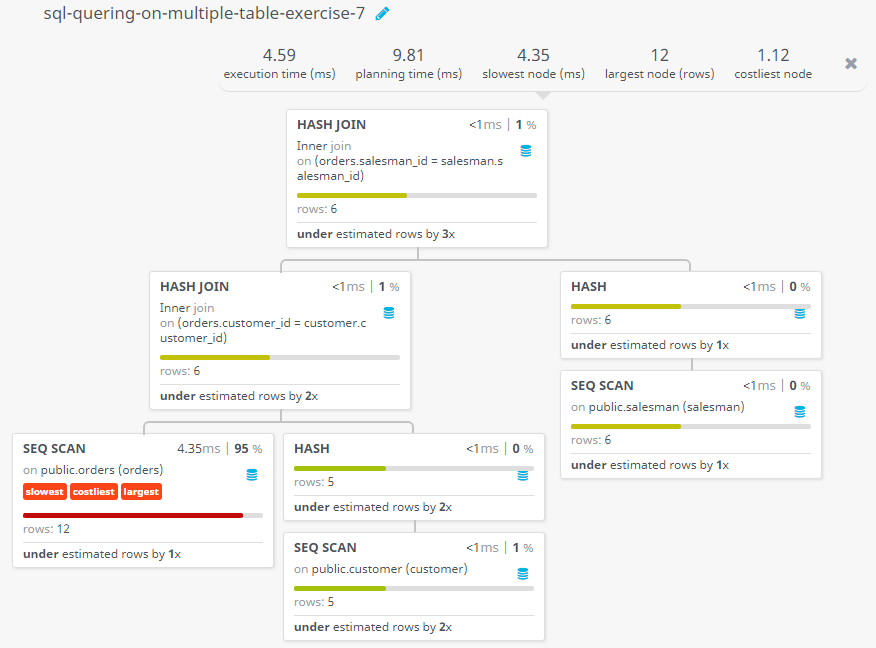
Cost:
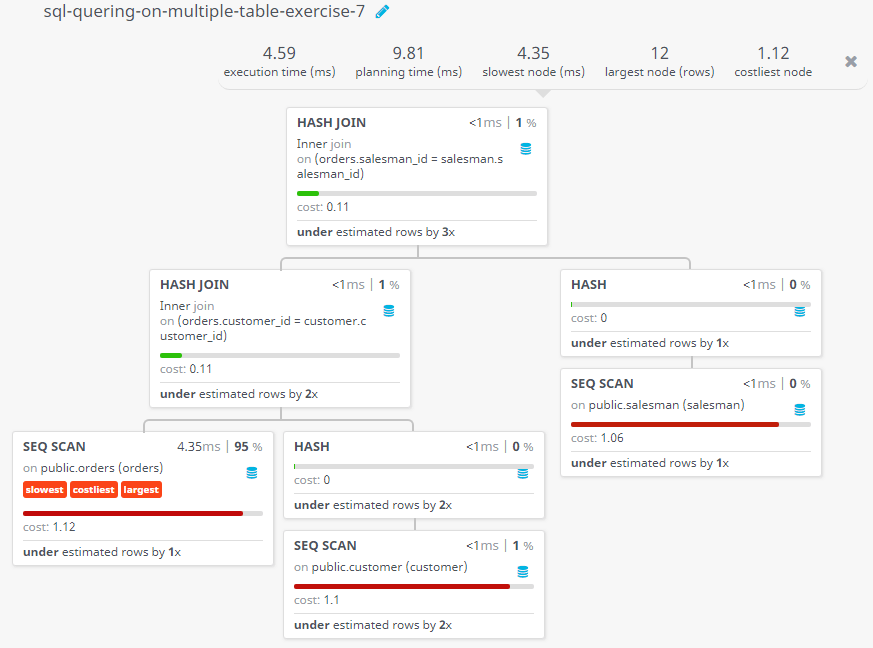
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Customers served by a salesman and commission.
Next SQL Exercise: Find all customers with orders on October 5, 2012.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics