SQL Exercise: Display commission of the salesman for an order
6. Order Details Report
From the following tables write a SQL query to find the details of an order. Return ord_no, ord_date, purch_amt, Customer Name, grade, Salesman, commission.
Sample table: orders
ord_no purch_amt ord_date customer_id salesman_id ---------- ---------- ---------- ----------- ----------- 70001 150.5 2012-10-05 3005 5002 70009 270.65 2012-09-10 3001 5005 70002 65.26 2012-10-05 3002 5001 70004 110.5 2012-08-17 3009 5003 70007 948.5 2012-09-10 3005 5002 70005 2400.6 2012-07-27 3007 5001 70008 5760 2012-09-10 3002 5001 70010 1983.43 2012-10-10 3004 5006 70003 2480.4 2012-10-10 3009 5003 70012 250.45 2012-06-27 3008 5002 70011 75.29 2012-08-17 3003 5007 70013 3045.6 2012-04-25 3002 5001
Sample table: customer
customer_id | cust_name | city | grade | salesman_id -------------+----------------+------------+-------+------------- 3002 | Nick Rimando | New York | 100 | 5001 3007 | Brad Davis | New York | 200 | 5001 3005 | Graham Zusi | California | 200 | 5002 3008 | Julian Green | London | 300 | 5002 3004 | Fabian Johnson | Paris | 300 | 5006 3009 | Geoff Cameron | Berlin | 100 | 5003 3003 | Jozy Altidor | Moscow | 200 | 5007 3001 | Brad Guzan | London | | 5005
Sample table: salesman
salesman_id | name | city | commission -------------+------------+----------+------------ 5001 | James Hoog | New York | 0.15 5002 | Nail Knite | Paris | 0.13 5005 | Pit Alex | London | 0.11 5006 | Mc Lyon | Paris | 0.14 5007 | Paul Adam | Rome | 0.13 5003 | Lauson Hen | San Jose | 0.12
Sample Solution:
-- Selecting specific columns and renaming them for clarity
SELECT a.ord_no, a.ord_date, a.purch_amt,
b.cust_name AS "Customer Name", b.grade,
c.name AS "Salesman", c.commission
-- Specifying the table to retrieve data from ('orders' as 'a')
FROM orders a
-- Performing an inner join with 'customer' table ('b') based on customer_id
INNER JOIN customer b
ON a.customer_id = b.customer_id
-- Performing another inner join with 'salesman' table ('c') based on salesman_id
INNER JOIN salesman c
ON a.salesman_id = c.salesman_id;
Output of the Query:
ord_no ord_date purch_amt Customer Name grade Salesman commission 70009 2012-09-10 270.65 Brad Guzan Pit Alex 0.11 70002 2012-10-05 65.26 Nick Rimando 100 James Hoog 0.15 70004 2012-08-17 110.50 Geoff Cameron 100 Lauson Hen 0.12 70005 2012-07-27 2400.60 Brad Davis 200 James Hoog 0.15 70008 2012-09-10 5760.00 Nick Rimando 100 James Hoog 0.15 70010 2012-10-10 1983.43 Fabian Johnson 300 Mc Lyon 0.14 70003 2012-10-10 2480.40 Geoff Cameron 100 Lauson Hen 0.12 70011 2012-08-17 75.29 Jozy Altidor 200 Paul Adam 0.13 70013 2012-04-25 3045.60 Nick Rimando 100 James Hoog 0.15 70001 2012-10-05 150.50 Graham Zusi 200 Nail Knite 0.13 70007 2012-09-10 948.50 Graham Zusi 200 Nail Knite 0.13 70012 2012-06-27 250.45 Julian Green 300 Nail Knite 0.13
Explanation:
The said SQL query that uses the INNER JOIN clause to combine rows from three tables: orders, customer, and salesman.
The query retrieves the ord_no, ord_date, purch_amt, cust_name, grade, name, and commission from the three tables where the customer_id from the orders table matches the customer_id from the customer table, and the salesman_id from the orders table matches the salesman_id from the salesman table.
The query also uses the AS keyword to rename the cust_name and name columns as 'Customer Name' and 'Salesman' respectively.
Visual Explanation:
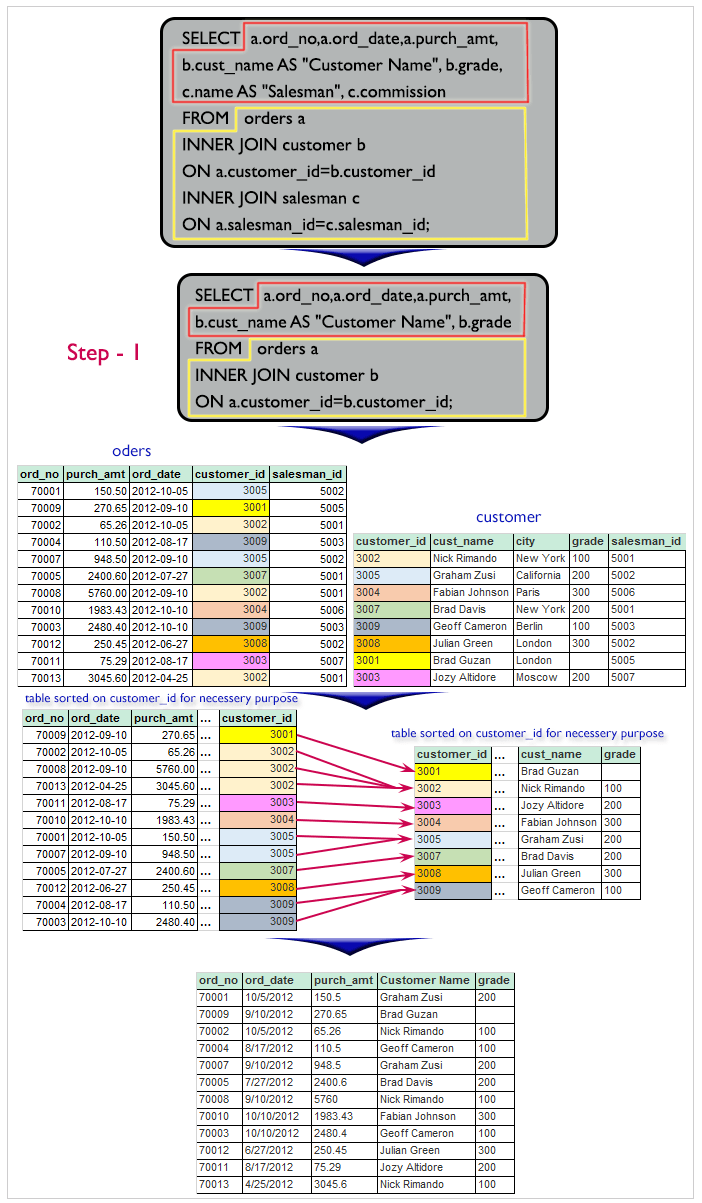
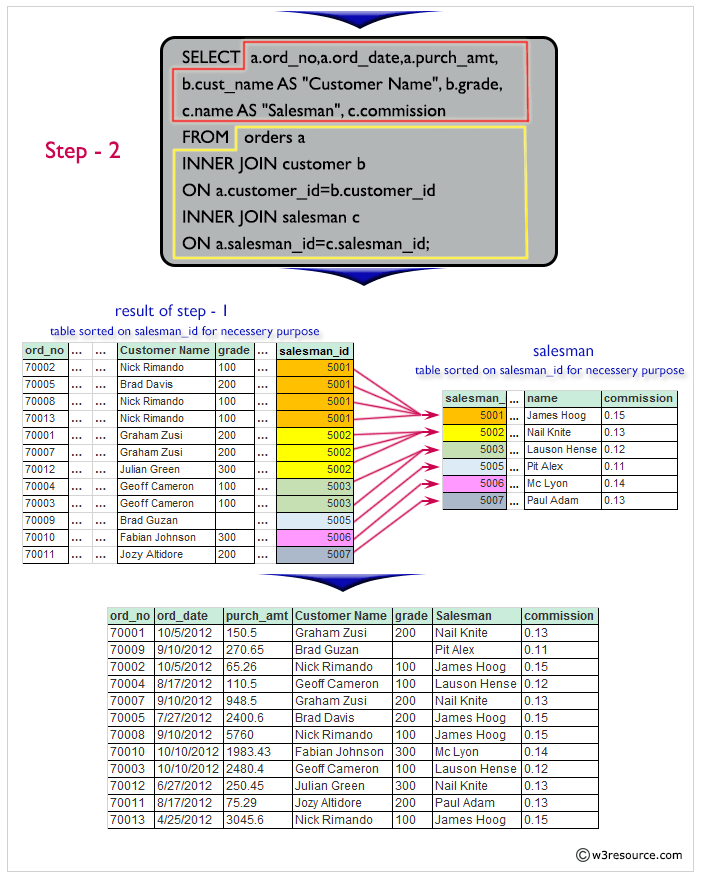
Practice Online
Query Visualization:
Duration:
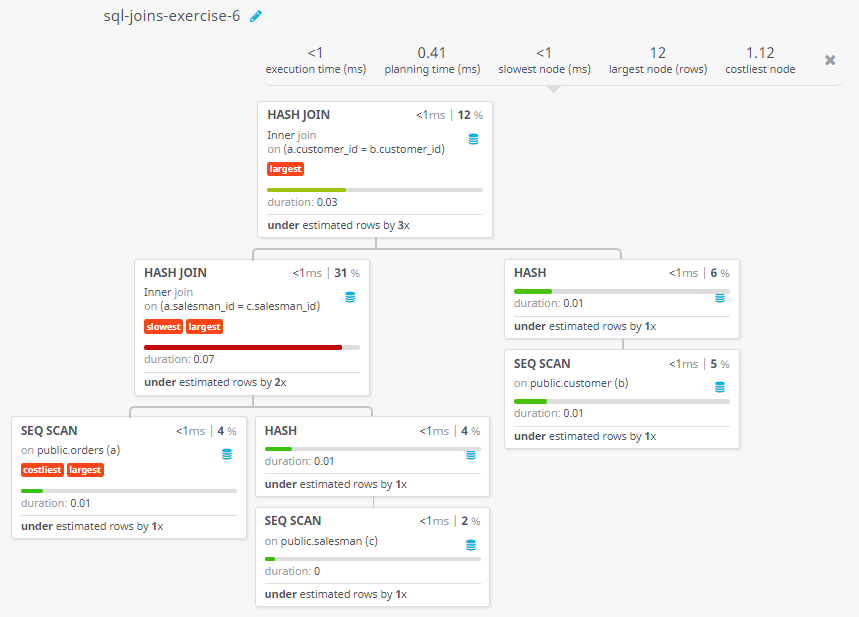
Rows:
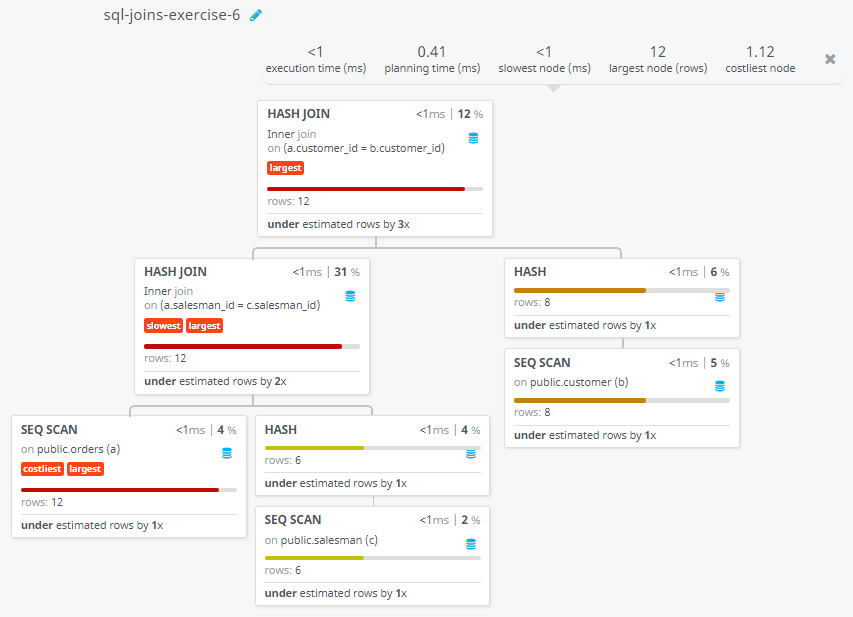
Cost:
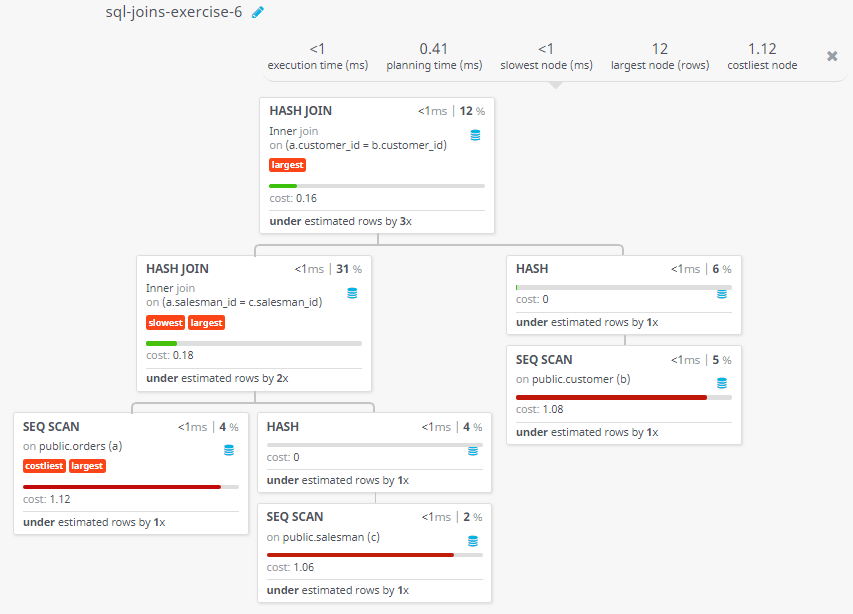
For more Practice: Solve these Related Problems:
- Write a SQL query to retrieve order number, order date, purchase amount, customer name, grade, salesperson name, and commission for each order.
- Write a SQL query to list full order details along with customer and salesperson information, ordering the result by order date.
- Write a SQL query to display detailed order information where orders with a purchase amount below 100 are filtered out.
- Write a SQL query to fetch order details along with customer and salesperson info, ensuring the order date is formatted as 'YYYY-MM-DD'.
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Customers and salespeople live in different cities.
Next SQL Exercise: Join within the tables salesman, customer and orders.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.