SQL Exercise: Customer have placed no order or one or more orders
Write a SQL statement to make a report with customer name, city, order number, order date, and order amount in ascending order according to the order date to determine whether any of the existing customers have placed an order or not.
Sample table: orders
ord_no purch_amt ord_date customer_id salesman_id ---------- ---------- ---------- ----------- ----------- 70001 150.5 2012-10-05 3005 5002 70009 270.65 2012-09-10 3001 5005 70002 65.26 2012-10-05 3002 5001 70004 110.5 2012-08-17 3009 5003 70007 948.5 2012-09-10 3005 5002 70005 2400.6 2012-07-27 3007 5001 70008 5760 2012-09-10 3002 5001 70010 1983.43 2012-10-10 3004 5006 70003 2480.4 2012-10-10 3009 5003 70012 250.45 2012-06-27 3008 5002 70011 75.29 2012-08-17 3003 5007 70013 3045.6 2012-04-25 3002 5001
Sample table: customer
customer_id | cust_name | city | grade | salesman_id -------------+----------------+------------+-------+------------- 3002 | Nick Rimando | New York | 100 | 5001 3007 | Brad Davis | New York | 200 | 5001 3005 | Graham Zusi | California | 200 | 5002 3008 | Julian Green | London | 300 | 5002 3004 | Fabian Johnson | Paris | 300 | 5006 3009 | Geoff Cameron | Berlin | 100 | 5003 3003 | Jozy Altidor | Moscow | 200 | 5007 3001 | Brad Guzan | London | | 5005
Sample Solution:
-- Selecting specific columns and renaming one column for clarity
SELECT a.cust_name, a.city, b.ord_no,
b.ord_date, b.purch_amt AS "Order Amount"
-- Specifying the tables to retrieve data from ('customer' as 'a' and 'orders' as 'b')
FROM customer a
-- Performing a left outer join based on the customer_id, including unmatched rows from 'customer'
LEFT OUTER JOIN orders b
ON a.customer_id = b.customer_id
-- Sorting the result set by ord_date in ascending order
ORDER BY b.ord_date;
Output of the Query:
cust_name city ord_no ord_date Order Amount Nick Rimando New York 70013 2012-04-25 3045.60 Julian Green London 70012 2012-06-27 250.45 Brad Davis New York 70005 2012-07-27 2400.60 Jozy Altidor Moscow 70011 2012-08-17 75.29 Geoff Cameron Berlin 70004 2012-08-17 110.50 Brad Guzan London 70009 2012-09-10 270.65 Nick Rimando New York 70008 2012-09-10 5760.00 Graham Zusi California 70007 2012-09-10 948.50 Graham Zusi California 70001 2012-10-05 150.50 Nick Rimando New York 70002 2012-10-05 65.26 Fabian Johnson Paris 70010 2012-10-10 1983.43 Geoff Cameron Berlin 70003 2012-10-10 2480.40
Explanation:
The said SQL query is selecting the customer name, city from the customer table aliased as a and the order number, order date and purchase amount from the orders table aliased as b. It is joining these tables on the 'customer_id' column, and the results are ordered by the 'ord_date' column.
Additionally, it is using a LEFT OUTER JOIN which will retrieve all records from the left table and the matching records from the right table. If no match is found on the right table, it will return NULL for the right table's fields.
Visual Explanation:
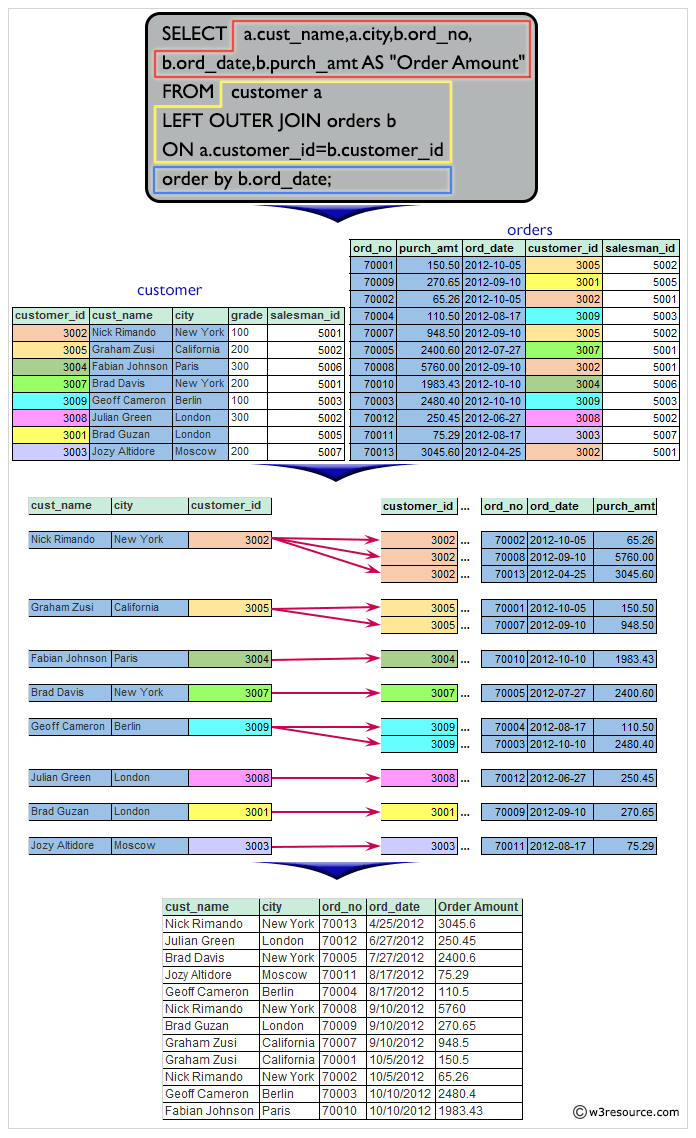
Practice Online
Query Visualization:
Duration:
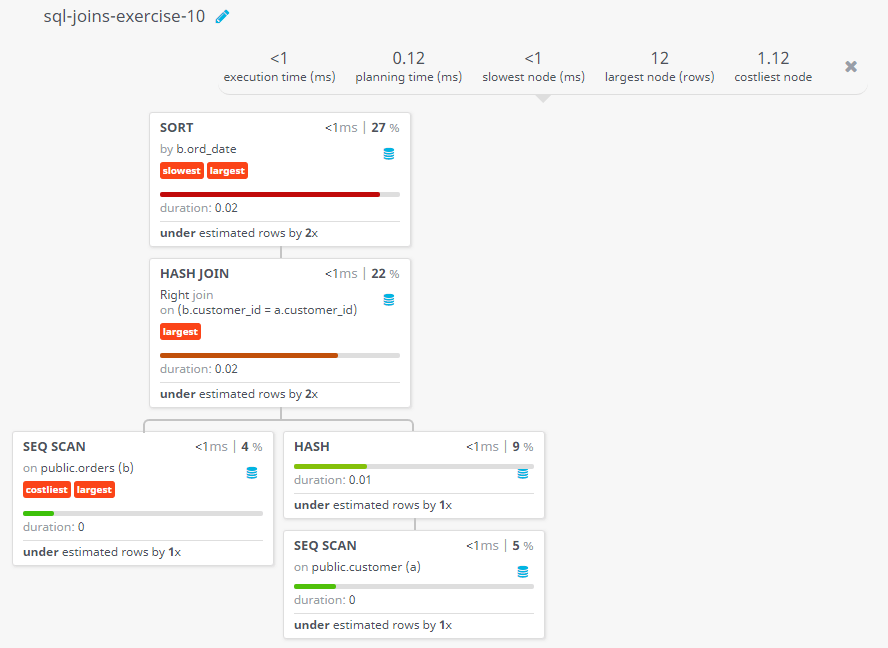
Rows:
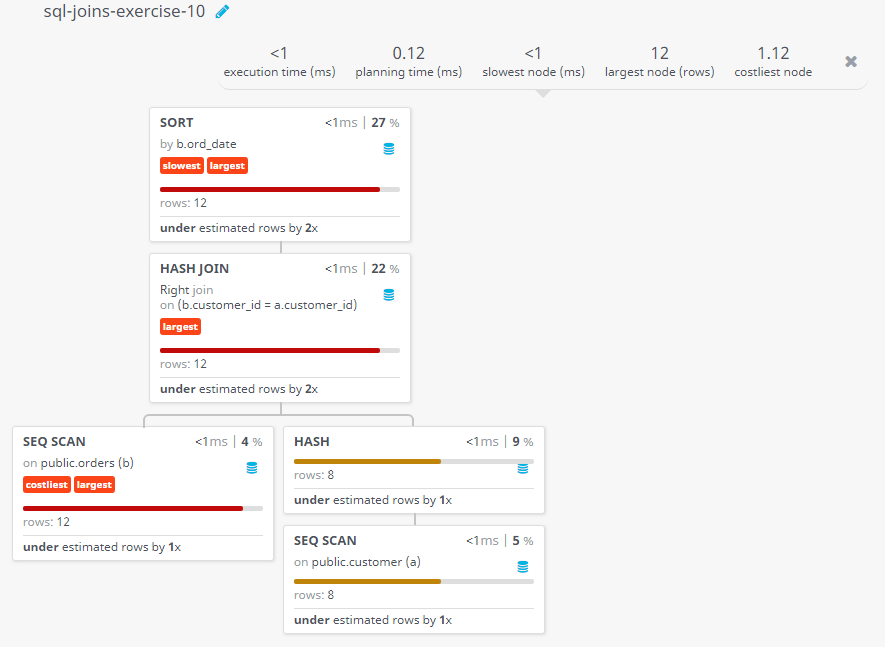
Cost:
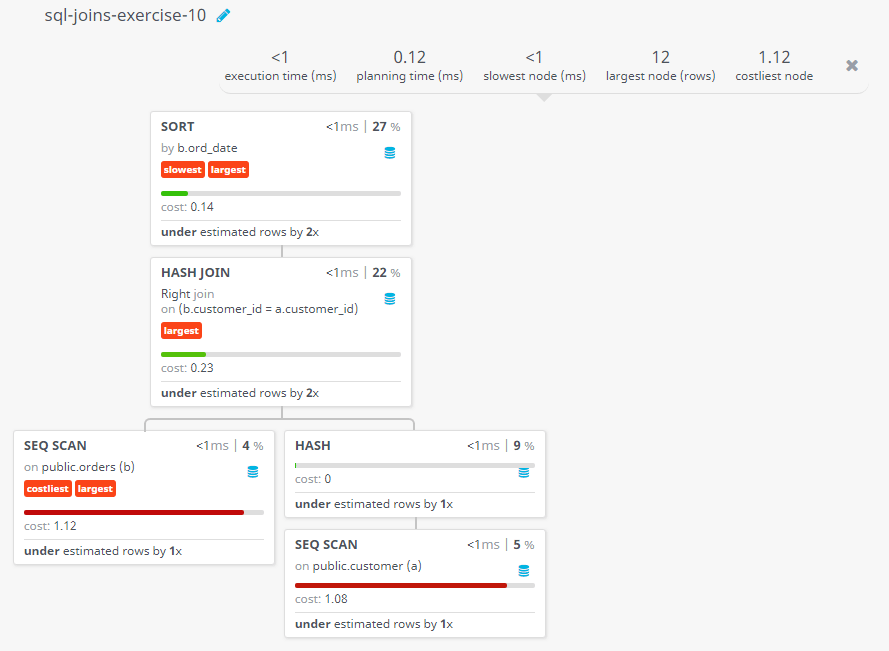
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Customers who holds a grade less than 300.
Next SQL Exercise: Find customers who have placed no order or one or more.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics