SQL Exercise: Doctors do the same procedure but are not certified
32. From the following tables, write a SQL query to find all physicians, their procedures, the date when the procedure was performed, and the name of the patient on whom the procedure was performed, but the physicians are not certified to perform that procedure. Return Physician Name as "Physician", Procedure Name as "Procedure", date, and Patient. Name as "Patient".
Sample table: physicianemployeeid | name | position | ssn ------------+-------------------+------------------------------+----------- 1 | John Dorian | Staff Internist | 111111111 2 | Elliot Reid | Attending Physician | 222222222 3 | Christopher Turk | Surgical Attending Physician | 333333333 4 | Percival Cox | Senior Attending Physician | 444444444 5 | Bob Kelso | Head Chief of Medicine | 555555555 6 | Todd Quinlan | Surgical Attending Physician | 666666666 7 | John Wen | Surgical Attending Physician | 777777777 8 | Keith Dudemeister | MD Resident | 888888888 9 | Molly Clock | Attending Psychiatrist | 999999999Sample table: undergoes
physician | treatment | certificationdate | certificationexpires -----------+-----------+-------------------+---------------------- 3 | 1 | 2008-01-01 | 2008-12-31 3 | 2 | 2008-01-01 | 2008-12-31 3 | 5 | 2008-01-01 | 2008-12-31 3 | 6 | 2008-01-01 | 2008-12-31 3 | 7 | 2008-01-01 | 2008-12-31 6 | 2 | 2008-01-01 | 2008-12-31 6 | 5 | 2007-01-01 | 2007-12-31 6 | 6 | 2008-01-01 | 2008-12-31 7 | 1 | 2008-01-01 | 2008-12-31 7 | 2 | 2008-01-01 | 2008-12-31 7 | 3 | 2008-01-01 | 2008-12-31 7 | 4 | 2008-01-01 | 2008-12-31 7 | 5 | 2008-01-01 | 2008-12-31 7 | 6 | 2008-01-01 | 2008-12-31 7 | 7 | 2008-01-01 | 2008-12-31Sample table: patient
ssn | name | address | phone | insuranceid | pcp -----------+-------------------+--------------------+----------+-------------+----- 100000001 | John Smith | 42 Foobar Lane | 555-0256 | 68476213 | 1 100000002 | Grace Ritchie | 37 Snafu Drive | 555-0512 | 36546321 | 2 100000003 | Random J. Patient | 101 Omgbbq Street | 555-1204 | 65465421 | 2 100000004 | Dennis Doe | 1100 Foobaz Avenue | 555-2048 | 68421879 | 3Sample table: procedure
code | name | cost ------+--------------------------------+------- 1 | Reverse Rhinopodoplasty | 1500 2 | Obtuse Pyloric Recombobulation | 3750 3 | Folded Demiophtalmectomy | 4500 4 | Complete Walletectomy | 10000 5 | Obfuscated Dermogastrotomy | 4899 6 | Reversible Pancreomyoplasty | 5600 7 | Follicular Demiectomy | 25Sample table: trained_in
physician | treatment | certificationdate | certificationexpires -----------+-----------+-------------------+---------------------- 3 | 1 | 2008-01-01 | 2008-12-31 3 | 2 | 2008-01-01 | 2008-12-31 3 | 5 | 2008-01-01 | 2008-12-31 3 | 6 | 2008-01-01 | 2008-12-31 3 | 7 | 2008-01-01 | 2008-12-31 6 | 2 | 2008-01-01 | 2008-12-31 6 | 5 | 2007-01-01 | 2007-12-31 6 | 6 | 2008-01-01 | 2008-12-31 7 | 1 | 2008-01-01 | 2008-12-31 7 | 2 | 2008-01-01 | 2008-12-31 7 | 3 | 2008-01-01 | 2008-12-31 7 | 4 | 2008-01-01 | 2008-12-31 7 | 5 | 2008-01-01 | 2008-12-31 7 | 6 | 2008-01-01 | 2008-12-31 7 | 7 | 2008-01-01 | 2008-12-31
Sample Solution:
SELECT p.name AS "Physician",
pr.name AS "Procedure",
u.date,
pt.name AS "Patient"
FROM physician p,
undergoes u,
patient pt,
PROCEDURE pr
WHERE u.patient = pt.SSN
AND u.procedure = pr.Code
AND u.physician = p.EmployeeID
AND NOT EXISTS
( SELECT *
FROM trained_in t
WHERE t.treatment = u.procedure
AND t.physician = u.physician );
Sample Output:
Physician | Procedure | date | Patient ------------------+-----------------------+---------------------+------------ Christopher Turk | Complete Walletectomy | 2008-05-13 00:00:00 | Dennis Doe (1 row)
Explanation:
The said query in SQL that retrieves the name of the physician, the procedure name and the date, and the name of the patient about procedures performed by physicians who have not been trained in that procedure.
The WHERE clause uses to join the tables 'undergoes' and 'patient' based on the patient and SSN columns, and the tables 'undergoes' and 'procedure' based on the procedure and code columns, and the tables 'undergoes' and 'physician' based on the physician and employee ID columns.
The NOT EXISTS keyword filters out the result of a subquery. The subquery searches the 'trained_in' table for a record with the same procedure code and physician ID as the current record in the 'undergoes' table. If a record is found, the subquery returns true and the current record is excluded from the result set.
Practice Online
E R Diagram of Hospital Database:
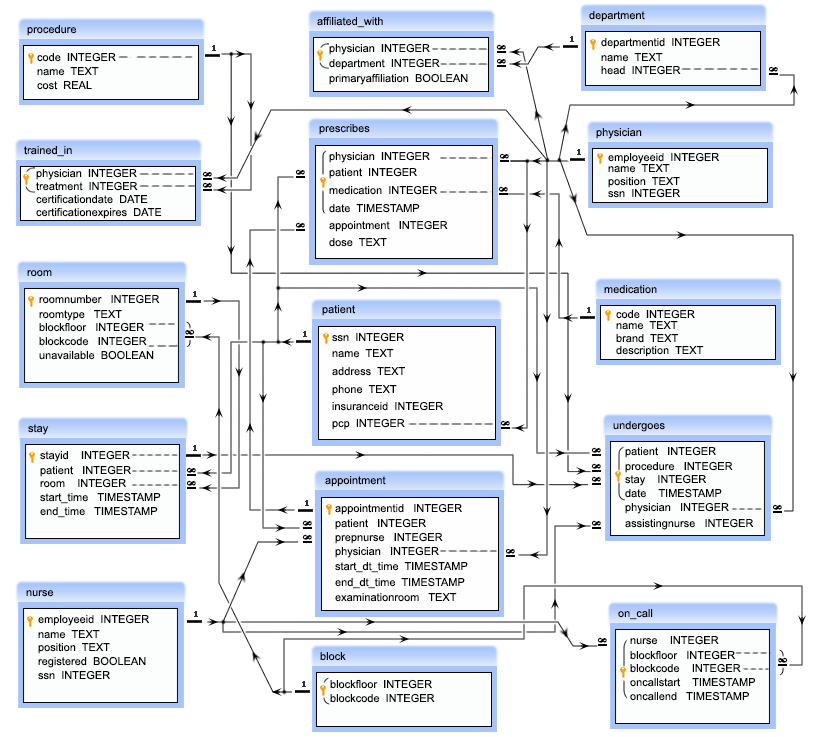
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Physicians, a medical procedure without certification.
Next SQL Exercise: Find all physicians who completed a medical procedure.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics