SQL Exercise: Find the floor with the minimum available rooms
27. From the following tables, write a SQL query to locate the floor with the minimum number of available rooms. Return floor ID as "Floor", Number of available rooms.
Sample table: roomroomnumber | roomtype | blockfloor | blockcode | unavailable -----------+----------+------------+-----------+------------- 101 | Single | 1 | 1 | f 102 | Single | 1 | 1 | f 103 | Single | 1 | 1 | f 111 | Single | 1 | 2 | f 112 | Single | 1 | 2 | t 113 | Single | 1 | 2 | f 121 | Single | 1 | 3 | f 122 | Single | 1 | 3 | f 123 | Single | 1 | 3 | f 201 | Single | 2 | 1 | t 202 | Single | 2 | 1 | f 203 | Single | 2 | 1 | f 211 | Single | 2 | 2 | f 212 | Single | 2 | 2 | f 213 | Single | 2 | 2 | t 221 | Single | 2 | 3 | f 222 | Single | 2 | 3 | f 223 | Single | 2 | 3 | f 301 | Single | 3 | 1 | f 302 | Single | 3 | 1 | t 303 | Single | 3 | 1 | f 311 | Single | 3 | 2 | f 312 | Single | 3 | 2 | f 313 | Single | 3 | 2 | f 321 | Single | 3 | 3 | t 322 | Single | 3 | 3 | f 323 | Single | 3 | 3 | f 401 | Single | 4 | 1 | f 402 | Single | 4 | 1 | t 403 | Single | 4 | 1 | f 411 | Single | 4 | 2 | f 412 | Single | 4 | 2 | f 413 | Single | 4 | 2 | f 421 | Single | 4 | 3 | t 422 | Single | 4 | 3 | f 423 | Single | 4 | 3 | f
Sample Solution:
SELECT blockfloor as "Floor",
count(*) AS "No of available rooms"
FROM room
WHERE unavailable='false'
GROUP BY blockfloor
HAVING count(*) =
(SELECT min(zz) AS highest_total
FROM
( SELECT blockfloor ,
count(*) AS zz
FROM room
WHERE unavailable='false'
GROUP BY blockfloor ) AS t );
Sample Output:
Floor | No of available rooms -------+----------------------- 3 | 7 4 | 7 2 | 7 (3 rows)
Explanation:
The said code in SQL that retrieves the number of available rooms on each floor of a building and only returns the floor(s) with the lowest number of available rooms.
The WHERE clause filters the rows in the room table where the unavailable column is equal to 'false', indicating that the room is available.
The GROUP BY clause groups the rows by the blockfloor column, so that the count function is applied to each distinct floor.
The subquery calculates the number of available rooms on each floor, and groups them by floor.
The MIN function in the outer query selects the lowest number of available rooms across all floors.
The HAVING clause compares the count of available rooms on each floor to the highest_total value returned by the subquery. Only the floors with the same count as the highest_total are returned.
Practice Online
E R Diagram of Hospital Database:
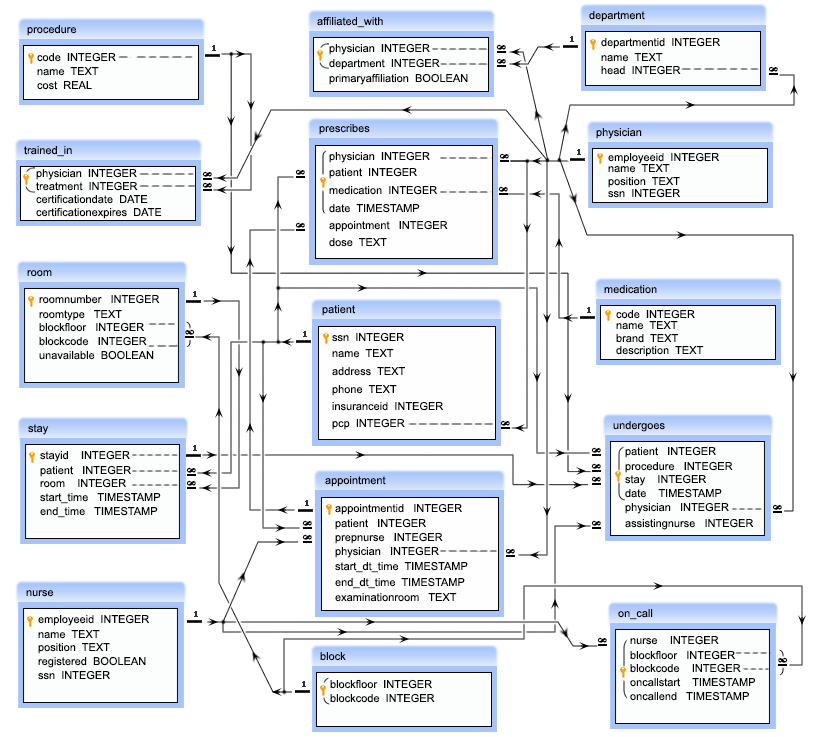
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Find the floor with the most rooms available.
Next SQL Exercise: Name of the patients, their block, floor, room number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics