SQL Exercise: Find the floor with the most rooms available
26. From the following tables, write a SQL query to find the floor where the maximum number of rooms are available. Return floor ID as "Floor", count "Number of available rooms".
Sample table: roomroomnumber | roomtype | blockfloor | blockcode | unavailable -----------+----------+------------+-----------+------------- 101 | Single | 1 | 1 | f 102 | Single | 1 | 1 | f 103 | Single | 1 | 1 | f 111 | Single | 1 | 2 | f 112 | Single | 1 | 2 | t 113 | Single | 1 | 2 | f 121 | Single | 1 | 3 | f 122 | Single | 1 | 3 | f 123 | Single | 1 | 3 | f 201 | Single | 2 | 1 | t 202 | Single | 2 | 1 | f 203 | Single | 2 | 1 | f 211 | Single | 2 | 2 | f 212 | Single | 2 | 2 | f 213 | Single | 2 | 2 | t 221 | Single | 2 | 3 | f 222 | Single | 2 | 3 | f 223 | Single | 2 | 3 | f 301 | Single | 3 | 1 | f 302 | Single | 3 | 1 | t 303 | Single | 3 | 1 | f 311 | Single | 3 | 2 | f 312 | Single | 3 | 2 | f 313 | Single | 3 | 2 | f 321 | Single | 3 | 3 | t 322 | Single | 3 | 3 | f 323 | Single | 3 | 3 | f 401 | Single | 4 | 1 | f 402 | Single | 4 | 1 | t 403 | Single | 4 | 1 | f 411 | Single | 4 | 2 | f 412 | Single | 4 | 2 | f 413 | Single | 4 | 2 | f 421 | Single | 4 | 3 | t 422 | Single | 4 | 3 | f 423 | Single | 4 | 3 | f
Sample Solution:
SELECT blockfloor as "Floor",
count(*) AS "Number of available rooms"
FROM room
WHERE unavailable='false'
GROUP BY blockfloor
HAVING count(*) =
(SELECT max(zz) AS highest_total
FROM
( SELECTblockfloor ,
count(*) AS zz
FROM room
WHERE unavailable='false'
GROUP BY blockfloor ) AS t );
Sample Output:
Floor | Number of available rooms -------+----------------------- 1 | 8 (1 row)
Explanation:
The given query in SQL that retrieves the floor and number of available rooms for each floor where rooms are available, groups the results by floor, and then returns only the floor(s) with the highest number of available rooms.
The WHERE clause filters the rows in the room table where the unavailable column is equal to 'false', indicating that the room is available.
The GROUP BY clause groups the rows by the blockfloor column, so that the count function is applied to each distinct floor.
The HAVING clause compares the count of available rooms on each floor to the highest_total value returned by the subquery. Only the floors with the same count as the highest_total are returned.
The subquery calculates the number of available rooms on each floor, and groups them by floor.
The MAX function in the outer query selects the highest number of available rooms across all floors.
Practice Online
E R Diagram of Hospital Database:
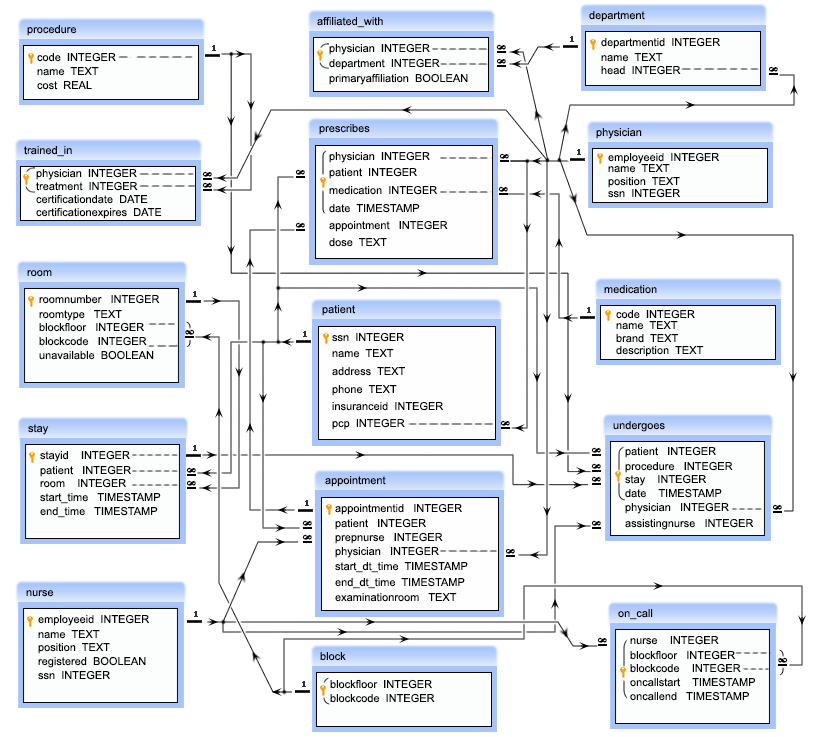
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Count unoccupied rooms in each block and floor.
Next SQL Exercise: Find the floor with the minimum available rooms.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.