R Programming: Create Inner, Outer, Left, and Right Joins
Write a R program to create inner, outer, left, right join(merge) from given two data frames.
Sample Solution :
R Programming Code :
# Create the first data frame with a column 'numid'
df1 = data.frame(numid = c(12, 14, 10, 11))
# Create the second data frame with a column 'numid'
df2 = data.frame(numid = c(13, 15, 11, 12))
# Print a message indicating the start of the Left Outer Join
print("Left outer Join:")
# Perform a Left Outer Join on 'numid' between df1 and df2
# This includes all rows from df1 and matched rows from df2
result = merge(df1, df2, by = "numid", all.x = TRUE)
# Print the result of the Left Outer Join
print(result)
# Print a message indicating the start of the Right Outer Join
print("Right outer Join:")
# Perform a Right Outer Join on 'numid' between df1 and df2
# This includes all rows from df2 and matched rows from df1
result = merge(df1, df2, by = "numid", all.y = TRUE)
# Print the result of the Right Outer Join
print(result)
# Print a message indicating the start of the Outer Join
print("Outer Join:")
# Perform a Full Outer Join on 'numid' between df1 and df2
# This includes all rows from both df1 and df2
result = merge(df1, df2, by = "numid", all = TRUE)
# Print the result of the Outer Join
print(result)
# Print a message indicating the start of the Cross Join
print("Cross Join:")
# Perform a Cross Join (Cartesian Product) between df1 and df2
# This includes all combinations of rows from both df1 and df2
result = merge(df1, df2, by = NULL)
# Print the result of the Cross Join
print(result)
Visual Presentation :
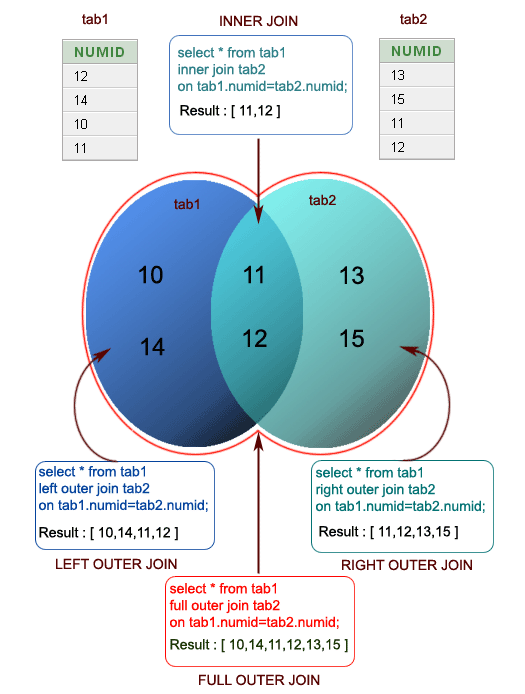
Output:
[1] "Left outer Join:" numid 1 10 2 11 3 12 4 14 [1] "Right outer Join:" numid 1 11 2 12 3 13 4 15 [1] "Outer Join:" numid 1 10 2 11 3 12 4 13 5 14 6 15 [1] "Cross Join:" numid.x numid.y 1 12 13 2 14 13 3 10 13 4 11 13 5 12 15 6 14 15 7 10 15 8 11 15 9 12 11 10 14 11 11 10 11 12 11 11 13 12 12 14 14 12 15 10 12 16 11 12
Explanation:
- Create Data Frames:
- df1 = data.frame(numid = c(12, 14, 10, 11))
- Creates the first data frame df1 with a column numid containing the values 12, 14, 10, and 11.
- df2 = data.frame(numid = c(13, 15, 11, 12))
- Creates the second data frame df2 with a column numid containing the values 13, 15, 11, and 12.
- Left Outer Join:
- print("Left outer Join:")
- Prints a message indicating that the following output will be from a Left Outer Join.
- result = merge(df1, df2, by = "numid", all.x = TRUE)
- Performs a Left Outer Join between df1 and df2 on the numid column, including all rows from df1 and matching rows from df2.
- print(result)
- Prints the result of the Left Outer Join.
- Right Outer Join:
- print("Right outer Join:")
- Prints a message indicating that the following output will be from a Right Outer Join.
- result = merge(df1, df2, by = "numid", all.y = TRUE)
- Performs a Right Outer Join between df1 and df2 on the numid column, including all rows from df2 and matching rows from df1.
- print(result)
- Prints the result of the Right Outer Join.
- Outer Join:
- print("Outer Join:")
- Prints a message indicating that the following output will be from a Full Outer Join.
- result = merge(df1, df2, by = "numid", all = TRUE)
- Performs a Full Outer Join between df1 and df2 on the numid column, including all rows from both df1 and df2.
- print(result)
- Prints the result of the Full Outer Join.
- Cross Join:
- print("Cross Join:")
- Prints a message indicating that the following output will be from a Cross Join (Cartesian Product).
- result = merge(df1, df2, by = NULL)
- Performs a Cross Join (Cartesian Product) between df1 and df2, which includes all combinations of rows from both data frames.
- print(result)
- Prints the result of the Cross Join.
R Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a R program to sort a given data frame by multiple column(s).
Next: Write a R program to replace NA values with 3 in a given data frame.
Test your Programming skills with w3resource's quiz.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics