Python unit test: Database connection validation
8. Unit Test for Database Connection Success
Write a Python unit test program to check if a database connection is successful.
Sample Solution:
Python Code:
# Import the 'unittest' module for writing unit tests.
import unittest
# Import the 'sqlite3' module for working with SQLite databases.
import sqlite3
# Define a test case class 'TestDatabaseConnection' that inherits from 'unittest.TestCase'.
class TestDatabaseConnection(unittest.TestCase):
# Define a test method 'test_database_connection' to test database connection.
def test_database_connection(self):
# Create a database connection in memory.
conn = sqlite3.connect(':memory:')
# Create a cursor to interact with the database.
cursor = conn.cursor()
# Execute a simple query to select the value 1.
cursor.execute("SELECT 1")
# Fetch the result of the query.
result = cursor.fetchone()
# Close the cursor and the database connection.
cursor.close()
conn.close()
# Assert that the fetched result is as expected, which should be a tuple containing 1.
self.assertEqual(result, (1,))
# Check if the script is run as the main program.
if __name__ == '__main__':
# Run the test cases using 'unittest.main()'.
unittest.main()
Sample Output:
Ran 1 test in 0.001s OK
Explanation:
The unittest module in Python can be used with a suitable database library such as sqlite3, psycopg2, or mysql-connector-python.
In the above exercise, the test_database_connection method tests if a database connection is successful. Inside the method, we create a database connection to an in-memory SQLite database using sqlite3.connect(':memory:'). We then create a cursor to execute SQL queries on the database.
In this case, we execute a simple query "SELECT 1" and fetch the result using cursor.fetchone(). The expected result is a tuple (1,).
At the end, we close both the cursor and the database connection by calling cursor.close() and conn.close().
Flowchart:
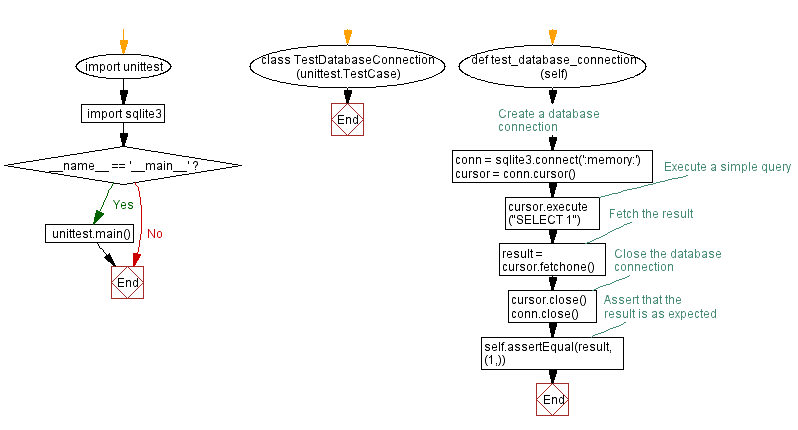
For more Practice: Solve these Related Problems:
- Write a Python unit test program to assert that a function returns a valid database connection object using a mock database.
- Write a Python unit test program to simulate a successful connection to a database and verify expected connection parameters.
- Write a Python unit test program to test error handling when attempting to connect to an invalid database URL.
- Write a Python unit test program to use unittest.mock to emulate a database connection and assert that the connection method is called correctly.
Python Code Editor :
Previous: Multi-threading handling verification.
Next: Database query result verification.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.