Python unit test: Multi-threading handling verification
Python Unit test: Exercise-7 with Solution
Write a Python unit test program to check if a function handles multi-threading correctly.
Sample Solution:
Python Code:
# Import the 'unittest' module for writing unit tests.
import unittest
# Import the 'threading' module to work with threads.
import threading
# Define a function 'perform_task' that simulates some computational task.
def perform_task():
# Initialize a result variable.
result = 0
# Simulate a task by summing numbers from 1 to 99999.
for i in range(1, 100000):
result += i
# Return the result of the task.
return result
# Define a test case class 'Test_Multi_Threading' that inherits from 'unittest.TestCase'.
class Test_Multi_Threading(unittest.TestCase):
# Define a test method 'test_multi_threading' to test multi-threading.
def test_multi_threading(self):
# Define the number of threads to create.
num_threads = 10
# Create an empty list to store thread objects.
threads = []
# Create and start multiple threads.
for _ in range(num_threads):
# Create a thread, specifying the target function 'perform_task'.
t = threading.Thread(target=perform_task)
# Append the thread to the 'threads' list.
threads.append(t)
# Start the thread's execution.
t.start()
# Wait for all threads to finish.
for t in threads:
t.join()
# Assert that all threads have completed successfully (not alive).
for t in threads:
self.assertFalse(t.is_alive())
# Check if the script is run as the main program.
if __name__ == '__main__':
# Run the test cases using 'unittest.main()'.
unittest.main()
Sample Output:
Ran 2 tests in 0.003s OK
Explanation:
In the above exercise -
The Test_Multi_Threading class inherits from unittest.TestCase. It contains a single test method test_multi_threading that tests if the perform_task function can be executed concurrently by multiple threads.
In the test_multi_threading method, we create a specified number of threads (num_threads) and add them to a list. We start each thread using the start method. Then, we wait for all threads to finish using the join method. Finally, we assert that all threads have completed by checking that none are still alive (is_alive method).
When running the unit test program, the unittest module executes the test_multi_threading method. The method will create multiple threads, start them, and wait for them to finish. All threads should complete successfully if the function handles multi-threading correctly, and the assertion self.assertFalse(t.is_alive()) will pass.
Flowchart:
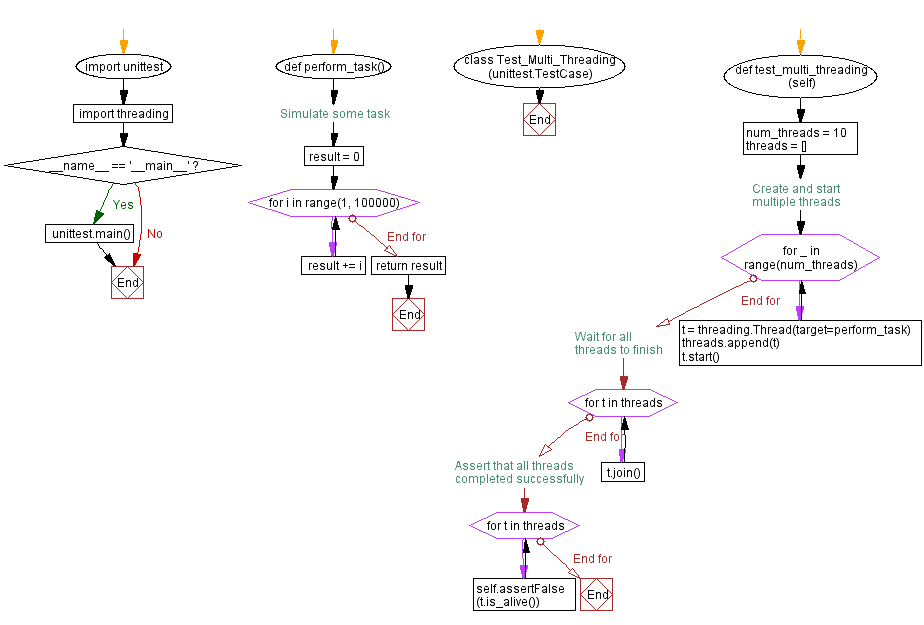
Previous: Accurate floating-point calculation handling.
Next: Database connection validation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics