Python unit test: Check palindrome string
4. Unit Test for Palindrome String Checker
Write a Python unit test program to check if a string is a palindrome.
Sample Solution:
Python Code:
# Import the 'unittest' module for writing unit tests.
import unittest
# Define a function 'is_palindrome' to check if a string is a palindrome.
def is_palindrome(string):
return string == string[::-1]
# Define a test case class 'TestPalindrome' that inherits from 'unittest.TestCase'.
class TestPalindrome(unittest.TestCase):
# Define a test method 'test_palindrome_string' to test palindrome strings.
def test_palindrome_string(self):
# Define a string 'palindrome' for testing palindrome or non-palindrome strings.
#palindrome = "madam"
palindrome = "hello"
print("Test palindrome:", palindrome)
# Assert that the string is a palindrome.
self.assertTrue(is_palindrome(palindrome), "The string is not a palindrome")
# Define a test method 'test_non_palindrome_string' to test non-palindrome strings.
def test_non_palindrome_string(self):
# Define a string 'non_palindrome' for testing palindrome or non-palindrome strings.
#non_palindrome = "hello"
non_palindrome = "madam"
print("Test non palindrome:", non_palindrome)
# Assert that the string is not a palindrome.
self.assertFalse(is_palindrome(non_palindrome), "The string is a palindrome")
# Check if the script is run as the main program.
if __name__ == '__main__':
# Run the test cases using 'unittest.main()'.
unittest.main()
Sample Output:
Ran 2 tests in 0.001s OK Test non palindrome: hello Test palindrome: madam
Ran 2 tests in 0.001s FAILED (failures=2) Test non palindrome: madam Test palindrome: hello
Explanation:
In the above exercise, we have a function is_palindrome(string) that takes a string as input and returns True if the string is a palindrome (reads the same forwards and backwards), and False otherwise. We define two test methods in the TestPalindrome class: test_palindrome_string and test_non_palindrome_string.
Flowchart:
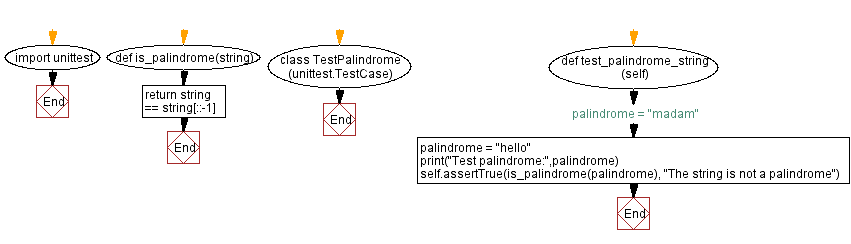
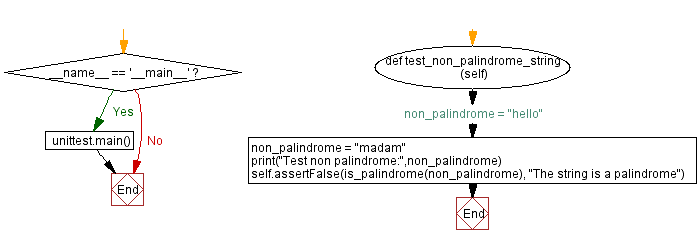
For more Practice: Solve these Related Problems:
- Write a Python unit test program to assert that a palindrome-checking function returns True for a simple palindromic string and False for a non-palindrome.
- Write a Python unit test program to test the palindrome function with mixed-case strings and verify that it is case-insensitive.
- Write a Python unit test program to check the behavior of the palindrome function for an empty string and a single-character string.
- Write a Python unit test program to test a function that identifies palindromic phrases by ignoring spaces and punctuation.
Go to:
Previous: Check equality of two lists.
Next: Check file existence in a directory.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.