Python unit test: Input data parsing and validation
Write a Python unit test program to check if a function correctly parses and validates input data.
Sample Solution:
Python Code:
# Import the 'unittest' module for writing unit tests.
import unittest
# Define a function 'parse_and_validate_input' for parsing and validating input data.
def parse_and_validate_input(data):
# Check if the input data is a string and contains only numeric characters.
if isinstance(data, str) and data.isnumeric():
# Convert the string to an integer and check if it's greater than 0.
return int(data) > 0
return False
# Define a test case class 'TestInputParsing' that inherits from 'unittest.TestCase'.
class TestInputParsing(unittest.TestCase):
# Define a test method 'test_valid_input' to test valid input data.
def test_valid_input(self):
# Define valid input data as a string containing a positive integer.
data = "100"
# Call the 'parse_and_validate_input' function with the valid input data.
result = parse_and_validate_input(data)
# Assert that the result is 'True' (indicating valid input).
self.assertTrue(result)
# Define a test method 'test_invalid_input' to test invalid input data.
def test_invalid_input(self):
# Define invalid input data as a string containing non-numeric characters.
data = "Hello"
# Call the 'parse_and_validate_input' function with the invalid input data.
result = parse_and validate_input(data)
# Assert that the result is 'False' (indicating invalid input).
self.assertFalse(result)
# Check if the script is run as the main program.
if __name__ == '__main__':
# Run the test cases using 'unittest.main()'.
unittest.main()
Sample Output:
Ran 2 tests in 0.001s OK
Explanation:
In the above exercise -
The parse_and_validate_input() function takes a data parameter and performs parsing and validation operations on it. The implementation details of this function are not shown, but you can customize it to fit your specific parsing and validation requirements.
The TestInputParsing class is a subclass of unittest.TestCase and contains test cases. We have two test cases: test_valid_input and test_invalid_input.
The parse_and_validate_input function takes a data parameter and performs the following checks:
- It checks if the data is a string using isinstance(data, str).
- It checks if the data is numeric by calling the isnumeric method on the string.
- It checks if the numerical value is greater than 0.
In the test_valid_input case, we provide valid input data "100", which satisfies all validation checks. Therefore, we expect the parse_and_validate_input function to return True, and the assertion self.assertTrue(result) will pass.
In the test_invalid_input case, we provide invalid input data "Hello", which fails the numeric check. Therefore, we expect the function to return False, and the assertion self.assertFalse(result) will pass.
Flowchart:
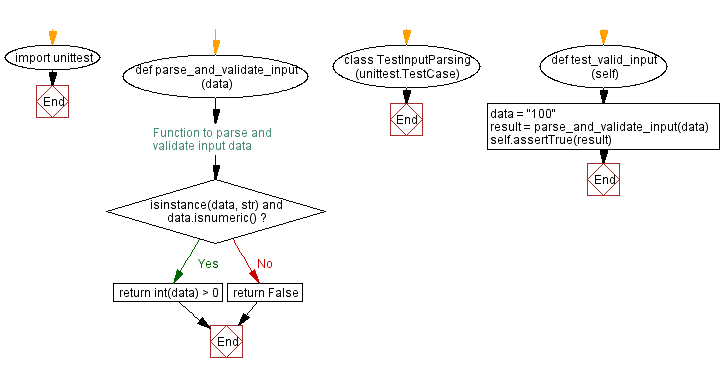
Previous: Database query result verification.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics