Python Exercise: Add an item in a tuple
5. Add an Item to a Tuple
Write a Python program to add an item to a tuple.
Visual Presentation:
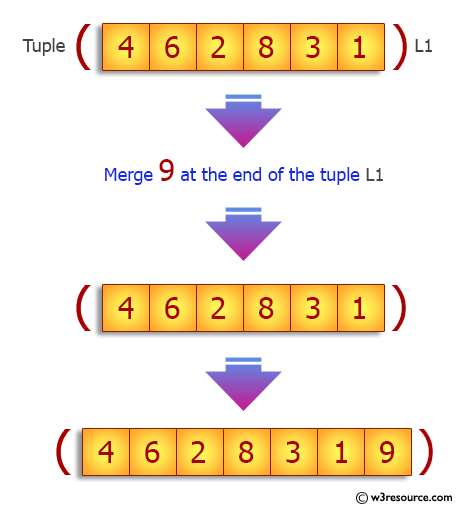
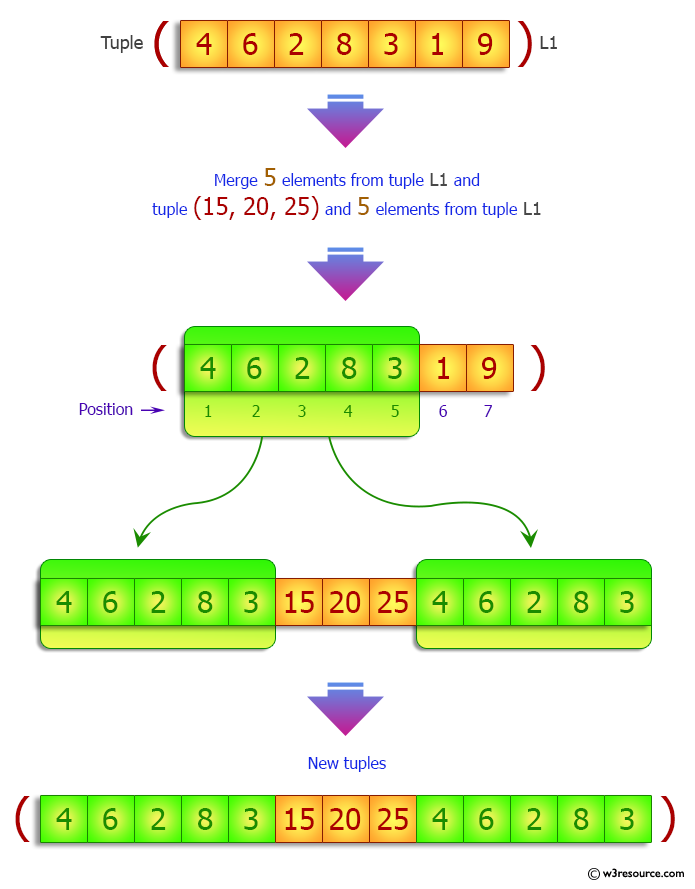
Sample Solution:
Python Code:
# Create a tuple containing a sequence of numbers
tuplex = (4, 6, 2, 8, 3, 1)
# Print the contents of the 'tuplex' tuple
print(tuplex)
# Tuples are immutable, so you can't add new elements directly.
# To add an element, create a new tuple by merging the existing tuple with the desired element using the + operator.
tuplex = tuplex + (9,)
# Print the updated 'tuplex' tuple
print(tuplex)
# Adding items at a specific index in the tuple.
# This line inserts elements (15, 20, 25) between the first five elements and duplicates the original five elements.
tuplex = tuplex[:5] + (15, 20, 25) + tuplex[:5]
# Print the modified 'tuplex' tuple
print(tuplex)
# Convert the tuple to a list.
listx = list(tuplex)
# Use different methods to add items to the list.
listx.append(30)
# Convert the modified list back to a tuple to obtain 'tuplex' with the added element.
tuplex = tuple(listx)
# Print the final 'tuplex' tuple with the added element
print(tuplex)
Sample Output:
(4, 6, 2, 8, 3, 1) (4, 6, 2, 8, 3, 1, 9) (4, 6, 2, 8, 3, 15, 20, 25, 4, 6, 2, 8, 3) (4, 6, 2, 8, 3, 15, 20, 25, 4, 6, 2, 8, 3, 30)
Flowchart:
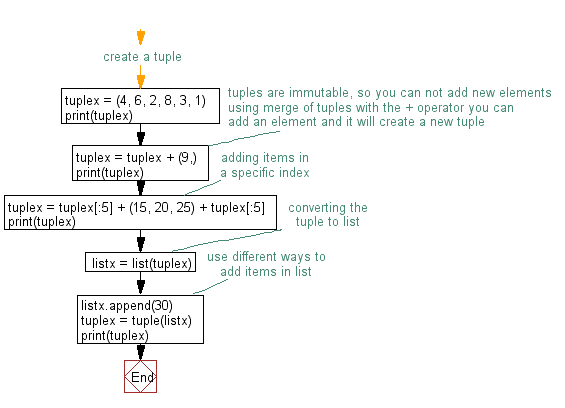
For more Practice: Solve these Related Problems:
- Write a Python program to add an element to an existing tuple by converting it to a list and then back to a tuple.
- Write a Python program to append multiple items to a tuple by concatenating tuples.
- Write a Python program to simulate insertion of an element at a specific index in a tuple.
- Write a Python program to create a function that takes a tuple and an element, and returns a new tuple with the element added at the end.
Python Code Editor:
Previous: Write a Python program to unpack a tuple in several variables.
Next: Write a Python program to convert a tuple to a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics