Python Exercise: Remove an item from a tuple
12. Remove an Item from a Tuple
Write a Python program to remove an item from a tuple.
Visual Presentation:
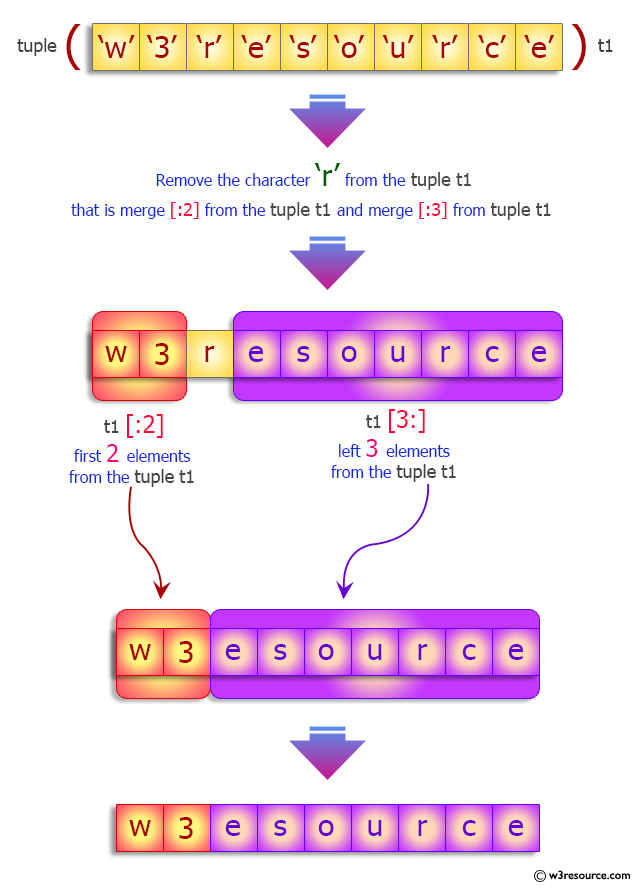
Sample Solution:
Python Code:
# Create a tuple containing a sequence of items
tuplex = "w", 3, "r", "s", "o", "u", "r", "c", "e"
# Print the contents of the 'tuplex' tuple
print(tuplex)
# Tuples are immutable, so you cannot remove elements directly.
# To "remove" an item, create a new tuple by merging the desired elements using the + operator.
tuplex = tuplex[:2] + tuplex[3:]
# Print the updated 'tuplex' tuple
print(tuplex)
# Convert the 'tuplex' tuple to a list
listx = list(tuplex)
# Use the 'remove' method to eliminate the item "c" from the list
listx.remove("c")
# Convert the modified list back to a tuple to obtain 'tuplex' with the item removed
tuplex = tuple(listx)
# Print the final 'tuplex' tuple
print(tuplex)
Sample Output:
('w', 3, 'r', 's', 'o', 'u', 'r', 'c', 'e') ('w', 3, 's', 'o', 'u', 'r', 'c', 'e') ('w', 3, 's', 'o', 'u', 'r', 'e')
Flowchart:
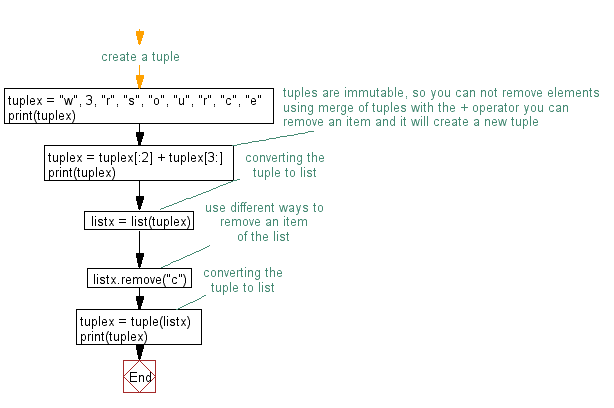
For more Practice: Solve these Related Problems:
- Write a Python program to remove a specified element from a tuple by converting it to a list and back to a tuple.
- Write a Python program to implement a function that returns a new tuple with a given element removed.
- Write a Python program to remove an element by its index from a tuple using slicing and concatenation.
- Write a Python program to simulate deletion from an immutable tuple by creating a new tuple that excludes the target element.
Python Code Editor:
Previous: Write a Python program to convert a list to a tuple.
Next: Write a Python program to slice a tuple.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.