Python tkinter widgets Exercise: Create a Progress bar widgets using tkinter module
Write a Python GUI program to create a Progress bar widgets using tkinter module.
Sample Solution:-
Python Code:
import tkinter as tk
from tkinter.ttk import Progressbar
from tkinter import ttk
parent = tk.Tk()
parent.title("Progressbar")
parent.geometry('350x200')
style = ttk.Style()
style.theme_use('default')
style.configure("black.Horizontal.TProgressbar", background='green')
bar = Progressbar(parent, length=220, style='black.Horizontal.TProgressbar')
bar['value'] = 80
bar.grid(column=0, row=0)
parent.mainloop()
Explanation:
In the exercise above -
- Import the Tkinter library (tkinter) and various modules for creating widgets and styles.
- Create the main Tkinter window (parent) with the title "Progressbar" and set its initial size to 350x200 pixels.
- style.configure("black.Horizontal.TProgressbar", background='green') - Define a custom style for the Progressbar using ttk.Style(). In this case, it's named "black.Horizontal.TProgressbar" and has a green background.
- bar = Progressbar(parent, length=220, style='black.Horizontal.TProgressbar') - Create a Progressbar widget (bar) with a specified length of 220 units and apply the custom style to it.
- bar['value'] = 80 - Set the value of the Progressbar to 80 (indicating 80% completion).
- bar.grid(column=0, row=0) - Use the grid() method to place the Progressbar within the window, specifying its position by column and row.
- parent.mainloop() - Start the Tkinter main loop to run the GUI application, displaying the Progressbar with the specified style and value.
Sample Output:
Flowchart:
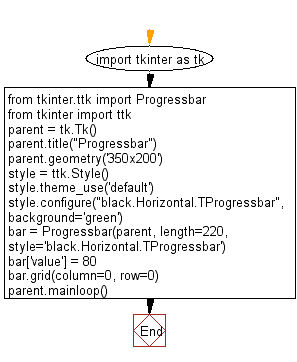
Go to:
Previous: Write a Python GUI program to create a ScrolledText widgets using tkinter module.
Next: Write a Python GUI program to create a Listbox bar widgets using tkinter module.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.