Python Tkinter Text Editor - Open and Save Text Files
Write a Python program that creates a text editor with Tkinter that opens a file dialog to open and edit text files. Implement event handling to save changes when a "Save" button is clicked.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import filedialog
# Function to open a text file and load its content into the Text widget
def open_file():
file_path = filedialog.askopenfilename(filetypes=[("Text Files", "*.txt")])
if file_path:
with open(file_path, "r") as file:
text_widget.delete("1.0", tk.END) # Clear the Text widget
text_widget.insert(tk.END, file.read()) # Insert file content
# Function to save the content of the Text widget to a text file
def save_file():
file_path = filedialog.asksaveasfilename(defaultextension=".txt", filetypes=[("Text Files", "*.txt")])
if file_path:
with open(file_path, "w") as file:
file.write(text_widget.get("1.0", tk.END)) # Write Text widget content to file
# Create the main window
root = tk.Tk()
root.title("Text Editor")
# Create a Text widget for text editing
text_widget = tk.Text(root, wrap=tk.WORD)
text_widget.pack(fill=tk.BOTH, expand=True)
# Create a "Open File" button to load a text file
open_button = tk.Button(root, text="Open File", command=open_file)
open_button.pack(side=tk.LEFT, padx=5, pady=5)
# Create a "Save" button to save changes to the file
save_button = tk.Button(root, text="Save", command=save_file)
save_button.pack(side=tk.LEFT, padx=5, pady=5)
# Start the Tkinter main loop
root.mainloop()
Explanation:
In the exercise above -
- Import the "tkinter" and "filedialog" modules.
- Define two functions:
- open_file: Opens a file dialog to select and open a text file. It reads the file's content and inserts it into the Text widget.
- save_file: Opens a file dialog to select a save location and saves the Text widget content to a text file.
- Create the main window and set its title.
- Create a Text widget (text_widget) for text editing, which allows text to be wrapped around word boundaries.
- Create an "Open File" button that, when clicked, calls the "open_file()" function to load a text file.
- Create a "Save" button that, when clicked, calls the "save_file()" function to save changes to the file. The 'defaultextension' option ensures that the file is saved with a ".txt" extension by default.
- Finally, start the Tkinter main loop with "root.mainloop()".
Output:
Flowchart:
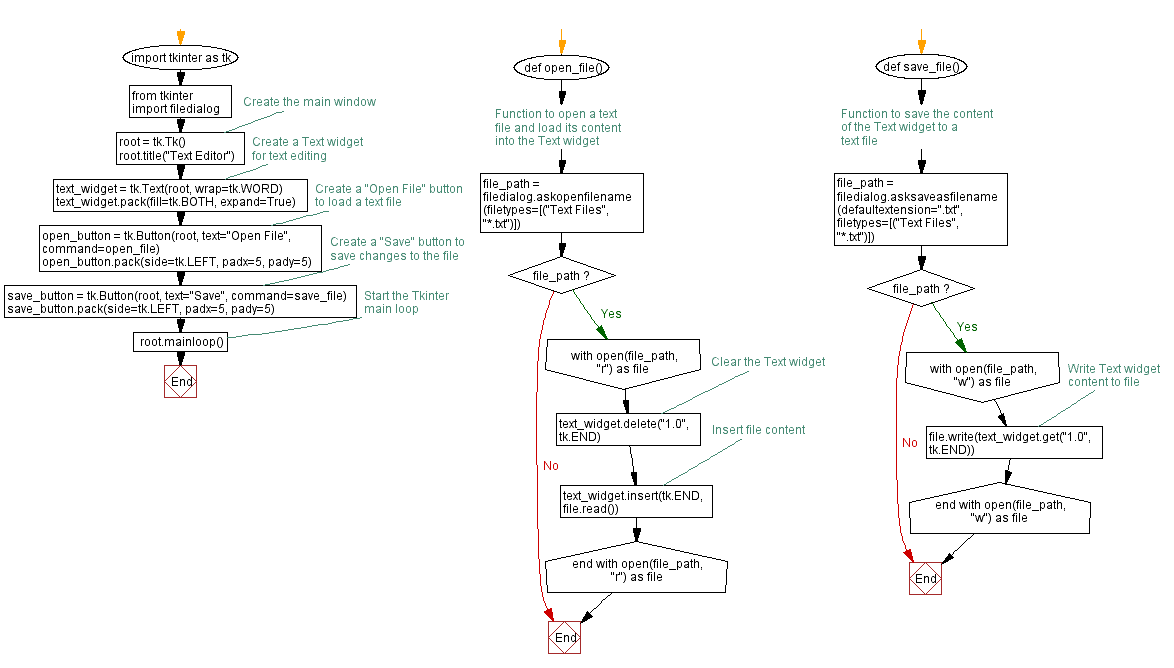
Go to:
Previous: Python Tkinter Canvas and Graphics Home.
Next: Freehand drawing and undo.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.