Python Tkinter file explorer - Browse and display folder contents
Write a Python program that implements a file explorer application using Tkinter. Allow users to select a folder, and when a folder is selected, display its contents in a Listbox.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import filedialog
import os
# Function to update the Listbox with folder contents
def browse_folder():
folder_path = filedialog.askdirectory() # Open a folder selection dialog
if folder_path:
folder_contents.delete(0, tk.END) # Clear the Listbox
for item in os.listdir(folder_path):
folder_contents.insert(tk.END, item) # Insert folder contents into Listbox
# Create the main window
root = tk.Tk()
root.title("File Explorer")
# Create a button to browse for a folder
browse_button = tk.Button(root, text="Browse Folder", command=browse_folder)
browse_button.pack(pady=10)
# Create a Listbox to display folder contents
folder_contents = tk.Listbox(root, selectmode=tk.SINGLE)
folder_contents.pack(fill=tk.BOTH, expand=True)
# Create a scrollbar for the Listbox
scrollbar = tk.Scrollbar(folder_contents, orient=tk.VERTICAL)
scrollbar.config(command=folder_contents.yview)
scrollbar.pack(side=tk.RIGHT, fill=tk.Y)
folder_contents.config(yscrollcommand=scrollbar.set)
# Start the Tkinter main loop
root.mainloop()
Explanation:
In the exercise above -
- Import the "tkinter" library and other necessary modules ("filedialog" and "os").
- Define a function "browse_folder()" that opens a folder selection dialog using "filedialog.askdirectory()". If a folder is selected, it clears the 'Listbox'(folder_contents) and populates it with the contents of the selected folder using "os.listdir".
- Create the main window and set its title.
- Create a "Browse Folder" button that, when clicked, calls the "browse_folder()" function to select a folder.
- Create a Listbox (folder_contents) to display the folder's contents, allowing single selection (selectmode=tk.SINGLE).
- Create a scrollbar for the Listbox and configure it to work with the Listbox's y-axis scrolling.
- Finally, start the Tkinter main loop with "root.mainloop()".
Output:
Flowchart:
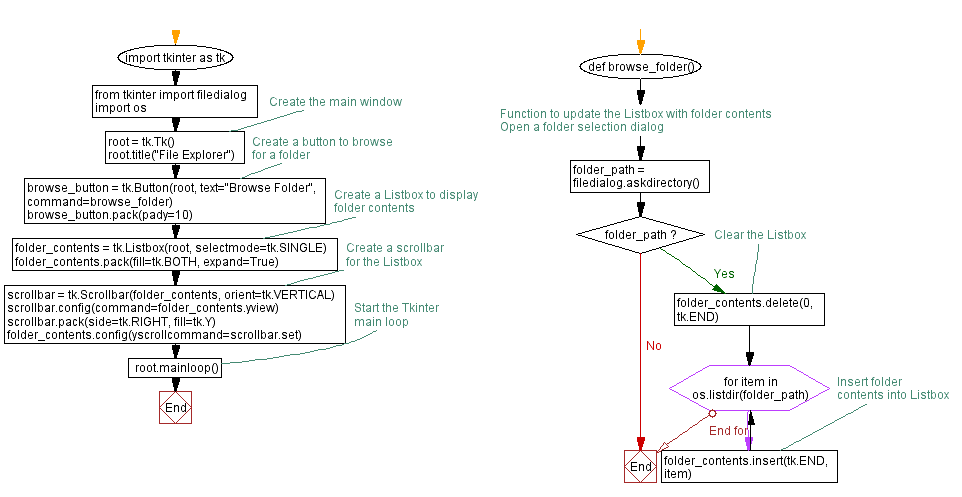
Go to:
Previous: Python Tkinter name input and message display.
Next: Open and Save Text Files.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.