Python Tkinter name input and message display
Python Tkinter Events and Event Handling: Exercise-6 with Solution
Write a Python program that allows users to input their name into a Tkinter Entry widget. When they press Enter or click a button, display a message with their name.
Sample Solution:
Python Code:
import tkinter as tk
# Function to display a message with the user's name
def display_name():
name = name_entry.get() # Get the text entered in the Entry widget
if name:
message_label.config(text=f"Hello, {name}!")
# Create the main window
root = tk.Tk()
root.title("Name Input")
# Create a Label widget for instructions
instruction_label = tk.Label(root, text="Input your name:")
instruction_label.pack(pady=10)
# Create an Entry widget for user input
name_entry = tk.Entry(root)
name_entry.pack(pady=5)
# Create a button to submit the name
submit_button = tk.Button(root, text="Submit", command=display_name)
submit_button.pack(pady=10)
# Create a Label widget to display the message
message_label = tk.Label(root, text="", font=("Helvetica", 16))
message_label.pack()
# Bind the Enter key to call the display_name function
root.bind("", lambda event=None: display_name())
# Start the Tkinter main loop
root.mainloop()
Explanation:
In the exercise above -
- Import the "tkinter" library.
- Define the “display_name()” function, which retrieves the text entered in the 'Entry' widget, and if a name is provided, it updates the 'message_label' with a message.
- Create the main window and set its title.
- Create a 'Label' widget (instruction_label) to provide instructions to the user.
- Create an 'Entry' widget (name_entry) where the user can input their name.
- Create a button (submit_button) with the label 'Submit' that, when clicked, calls the "display_name()" function.
- Create a Label widget (message_label) that initially displays an empty message but updates with the user's name when submitted.
- We bind the 'Enter' key to call the "display_name()" function, allowing the user to press Enter after entering their name.
- Finally, start the Tkinter main loop with "root.mainloop()".
Output:
Flowchart:
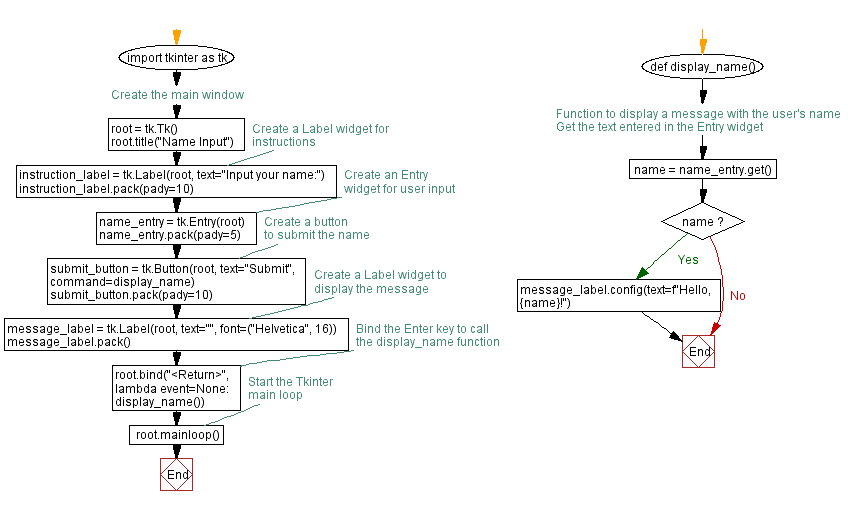
Python Code Editor:
Previous: Python Tkinter Color Picker Example.
Next: Browse and display folder contents.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/tkinter/python-tkinter-events-and-event-handling-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics