Python Tkinter Listbox example with event handling
Write a Python program that displays a list of items in a Tkinter Listbox. Implement an event handler to print the selected item when a button is clicked.
Sample Solution:
Python Code:
import tkinter as tk
def print_selected_item():
selected_index = listbox.curselection() # Get the selected item's index
if selected_index:
selected_item = listbox.get(selected_index[0]) # Get the selected item
result_label.config(text=f"Selected Item: {selected_item}")
else:
result_label.config(text="No item selected")
# Create the main window
root = tk.Tk()
root.title("Listbox Example")
# Create a Listbox widget and populate it with items
listbox = tk.Listbox(root)
listbox.pack(pady=10)
items = ["Java Exercises", "c++ Exercises", "C# Exercises", "C# Exercises", "Python Exercises"]
for item in items:
listbox.insert(tk.END, item)
# Create a button to print the selected item
print_button = tk.Button(root, text="Print Selected Item", command=print_selected_item)
print_button.pack()
# Create a label to display the selected item
result_label = tk.Label(root, text="")
result_label.pack()
# Start the Tkinter main loop
root.mainloop()
Explanation:
In the exercise above -
- Import the "tkinter" module.
- Create a Tkinter window and a Listbox widget to display a list of items.
- Populate the "Listbox" with some sample items.
- Create a button labeled "Print Selected Item" and associate it with the "print_selected_item()" function using the command parameter.
- When the button is clicked, the "print_selected_item()" function is called.
- In the "print_selected_item()" function, we first get the index of the selected item using listbox.curselection(). If an item is selected, we retrieve the selected item using listbox.get(selected_index[0]).
- Display a message if no item has been selected or update a label (result_label) with the selected item's text.
- Finally, start the Tkinter main loop with root.mainloop()
Output:
Flowchart:
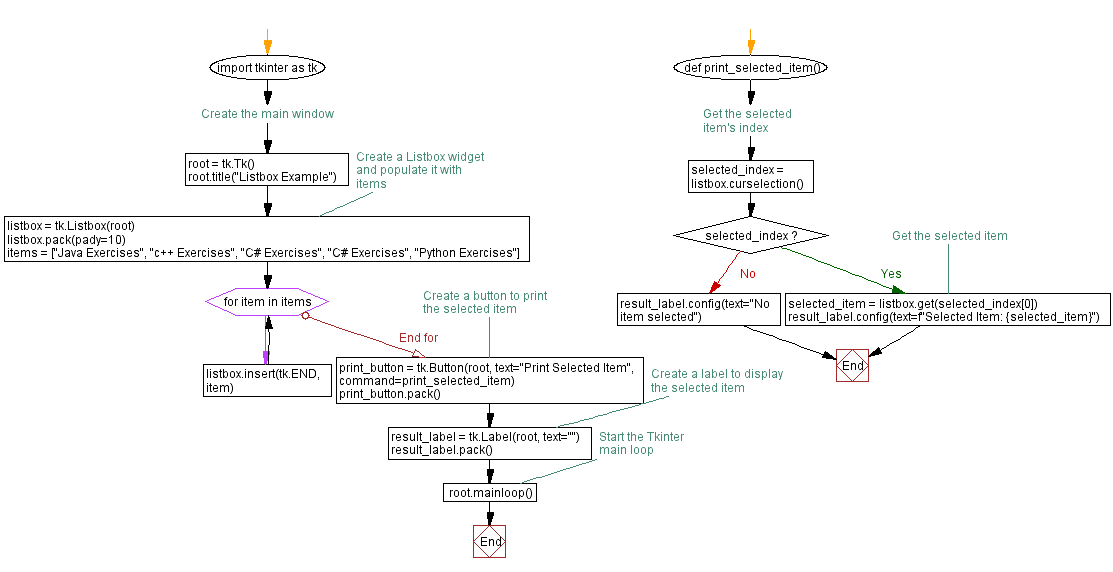
Python Code Editor:
Previous: Python Tkinter simple calculator example.
Next: Python Tkinter Color Picker Example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics