Python Tkinter font size adjuster - Scale widget example
Write a Python program to build a Tkinter scale widget that adjusts the font size of text displayed in a Label widget as the scale value changes.
Sample Solution:
Python Code:
import tkinter as tk
class FontSizeAdjuster:
def __init__(self, root):
self.root = root
self.root.title("Font Size Adjuster")
# Create a Label to display text with adjustable font size
self.label = tk.Label(root, text="Python-Tkinter", font=("Helvetica", 12))
self.label.pack(pady=20)
# Create a Scale widget to adjust font size
self.scale = tk.Scale(root, from_=8, to=36, orient=tk.HORIZONTAL, label="Font Size",
command=self.update_font_size)
self.scale.pack()
def update_font_size(self, font_size):
# Update the font size of the Label text
self.label.config(font=("Helvetica", int(font_size)))
if __name__ == "__main__":
root = tk.Tk()
app = FontSizeAdjuster(root)
root.mainloop()
Explanation:
In the exercise above -
- Import the "tkinter" library.
- Create a FontSizeAdjuster class that encapsulates the application.
- Inside the init method of the class, we create a Label widget (self.label) to display the text with an adjustable font size. We set the initial font size to '12'.
- Create a Scale widget (self.scale) that allows the user to adjust the font size. It ranges from '8' to '36' with a horizontal orientation. The command parameter is set to the "update_font_size()" method, which will be called whenever the scale value changes.
- The "update_font_size()" method is called whenever the user changes the scale value. It takes the selected font size as an argument and updates the font of the Label widget to reflect the chosen font size.
- In the if name == "__main__": block, we create the main Tkinter window, initialize the FontSizeAdjuster app, and start the Tkinter main event loop to run the application.
Output:
Flowchart:
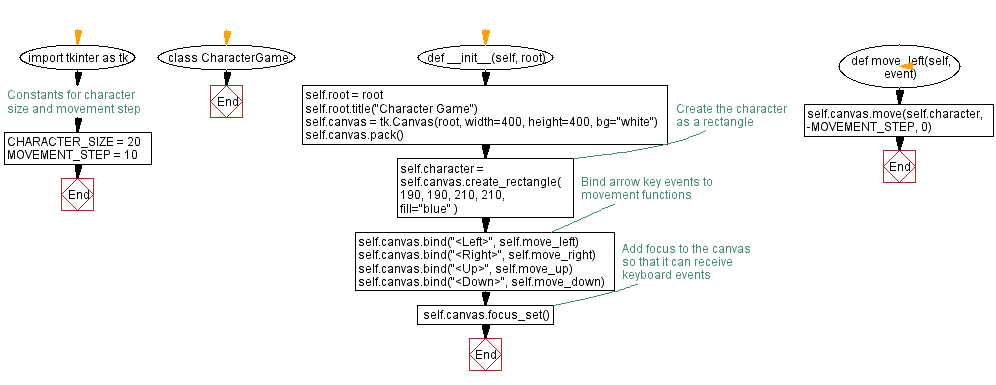
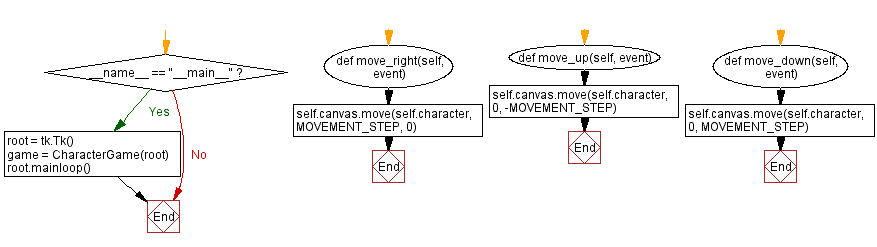
Python Code Editor:
Previous: Character movement example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics