Python Tkinter arrow Key game - Character movement example
Write a Python program to create a simple game using Tkinter where a character moves on arrow key presses. Implement event handling for left, right, up, and down arrow keys.
Sample Solution:
Python Code:
import tkinter as tk
# Constants for character size and movement step
CHARACTER_SIZE = 20
MOVEMENT_STEP = 10
class CharacterGame:
def __init__(self, root):
self.root = root
self.root.title("Character Game")
self.canvas = tk.Canvas(root, width=400, height=400, bg="white")
self.canvas.pack()
# Create the character as a rectangle
self.character = self.canvas.create_rectangle(
190, 190, 210, 210, fill="blue"
)
# Bind arrow key events to movement functions
self.canvas.bind("<Left>", self.move_left)
self.canvas.bind("<Right>", self.move_right)
self.canvas.bind("<Up>", self.move_up)
self.canvas.bind("<Down>", self.move_down)
# Add focus to the canvas so that it can receive keyboard events
self.canvas.focus_set()
def move_left(self, event):
self.canvas.move(self.character, -MOVEMENT_STEP, 0)
def move_right(self, event):
self.canvas.move(self.character, MOVEMENT_STEP, 0)
def move_up(self, event):
self.canvas.move(self.character, 0, -MOVEMENT_STEP)
def move_down(self, event):
self.canvas.move(self.character, 0, MOVEMENT_STEP)
if __name__ == "__main__":
root = tk.Tk()
game = CharacterGame(root)
root.mainloop()
Explanation:
In the exercise above -
- Import the "tkinter" library.
- Define constants:
- CHARACTER_SIZE = 20: This constant defines the size of the character (rectangle) in pixels.
- MOVEMENT_STEP = 10: This constant defines the step size for each movement when an arrow key is pressed.
- Create a class "CharacterGame" which encapsulates the entire game.
- Initialize the game:
- In the init method, the game initializes by creating the main window, setting its title, and creating a canvas widget for drawing the character.
- self.root stores the main Tkinter window.
- self.canvas is a canvas widget created within the main window where the character will be displayed.
- self.character represents the character (a blue rectangle) drawn on the canvas.
- Bind arrow key events to movement functions:
- self.canvas.bind("<Left>", self.move_left): Binds the left arrow key event to the move_left method.
- self.canvas.bind("<Right>", self.move_right): Binds the right arrow key event to the move_right method.
- self.canvas.bind("<Up>", self.move_up): Binds the up arrow key event to the move_up method.
- self.canvas.bind("<Down>", self.move_down): Binds the down arrow key event to the move_down method.
- Add focus to the canvas:
- self.canvas.focus_set(): This line ensures that the canvas receives keyboard focus, allowing it to respond to arrow key presses.
- Define movement methods:
- move_left, move_right, move_up, and move_down are methods that move the character within the canvas based on the arrow key pressed. They use the self.canvas.move method to change the character's position.
- Create an instance of the CharacterGame class:
- root = tk.Tk(): Creates the main Tkinter window.
- game = CharacterGame(root): Creates an instance of the CharacterGame class, which initializes the game.
- root.mainloop(): Starts the Tkinter main event loop, allowing the game to run and respond to user input.
- Finally, start the Tkinter main loop with "root.mainloop()".
Output:
Flowchart:
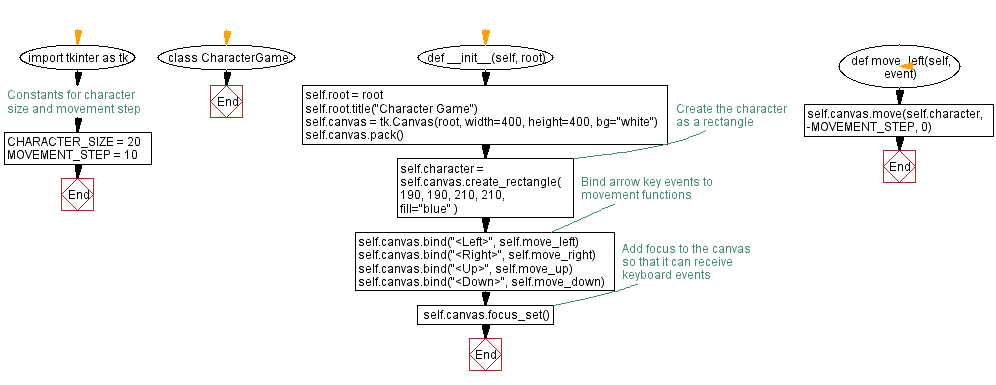
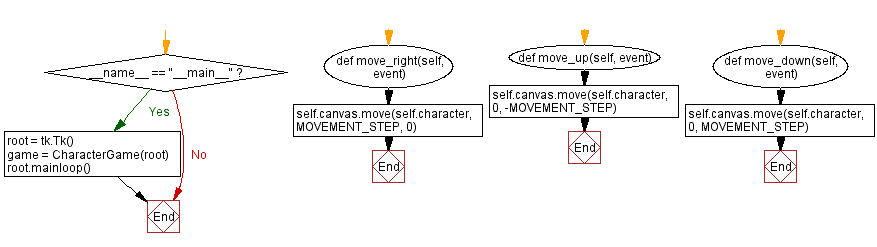
Python Code Editor:
Previous: Create menu options.
Next: Scale widget example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics