Python Tkinter message box example
Write a Python program that displays a button using Tkinter. When the button is clicked, display a message box with the message.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import messagebox
# Function to display the message box
def show_message_box():
messagebox.showinfo("Message", "Button Clicked!")
# Create the main window
root = tk.Tk()
root.title("Message Box Example")
# Create a button widget
button = tk.Button(root, text="Click here to display a message", command=show_message_box)
button.pack(padx=20, pady=20)
# Start the Tkinter main loop
root.mainloop()
Explanation:
In the exercise above -
- Import the necessary modules, including tkinter and messagebox.
- Define a function "show_message_box()" function that displays the message box using messagebox.showinfo() when called.
- Create the main Tkinter window 'root' and set its title.
- Create a button widget labeled "Click here to display a message" and associate it with the "show_message_box()" function using the command parameter.
- Finally, start the Tkinter main loop with “root.mainloop()”, which keeps the GUI application running.
Output:
Flowchart:
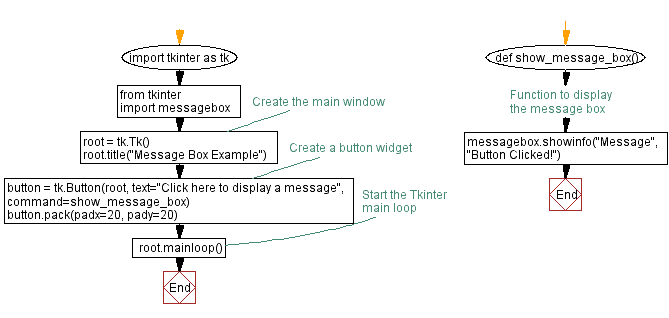
Python Code Editor:
Previous: Python Tkinter Events and Event Handling Home.
Next: Python Tkinter label and button example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics