Python Tkinter text editor with file dialogs
Write a Python program using Tkinter to build a text editor application that combines file open, save, and save as dialogs for editing text files.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import filedialog
def open_file():
file_path = filedialog.askopenfilename(title="Open Text File", filetypes=[("Text files", "*.txt"), ("All files", "*.*")])
if file_path:
with open(file_path, 'r') as file:
text_widget.delete("1.0", tk.END)
text_widget.insert(tk.END, file.read())
status_label.config(text=f"File opened: {file_path}")
def save_file():
file_path = current_file.get()
if file_path:
try:
with open(file_path, 'w') as file:
text_content = text_widget.get("1.0", tk.END)
file.write(text_content)
status_label.config(text=f"File saved: {file_path}")
except Exception as e:
status_label.config(text=f"Error saving file: {str(e)}")
else:
save_as_file()
def save_as_file():
file_path = filedialog.asksaveasfilename(defaultextension=".txt", filetypes=[("Text files", "*.txt"), ("All files", "*.*")])
if file_path:
current_file.set(file_path)
save_file()
root = tk.Tk()
root.title("Text Editor")
current_file = tk.StringVar()
menu_bar = tk.Menu(root)
root.config(menu=menu_bar)
file_menu = tk.Menu(menu_bar, tearoff=0)
menu_bar.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="Open", command=open_file)
file_menu.add_command(label="Save", command=save_file)
file_menu.add_command(label="Save As", command=save_as_file)
file_menu.add_separator()
file_menu.add_command(label="Exit", command=root.destroy)
text_widget = tk.Text(root, wrap=tk.WORD)
text_widget.pack(padx=20, pady=20, fill="both", expand=True)
status_label = tk.Label(root, text="", padx=20, pady=10)
status_label.pack()
root.mainloop()
Explanation:
In the exercise above -
- Import "tkinter" as "tk" and "filedialog" for creating GUI components and opening file dialogs.
- Define the "open_file()" function, which displays a file dialog using "filedialog.askopenfilename()" function. This dialog allows the user to select a text file to open. If a file is selected, it reads the file content and displays it in the Text widget. The status label shows the opened file path.
- Define the save_file() function, which saves the current Text widget content to the current file path. If there is no current file path, it calls the "save_as_file()" function to specify a new file path. The status label shows messages about file saving or errors.
- Define the "save_as_file()" function, which opens a file save dialog using "filedialog.asksaveasfilename()" function. This dialog allows the user to specify a file path for saving the text. If a file path is selected, it sets the current file path and calls the "save_file()" function to save the content.
- Create the main Tkinter window, set its title, and create a Text widget for editing text.
- Create a StringVar variable current_file to keep track of the currently opened or saved file.
- Create a menu bar with a "File" menu that contains options for opening, saving, saving as, and exiting the program.
- The main event loop, "root.mainloop()", starts the Tkinter application.
Sample Output:
Flowchart:
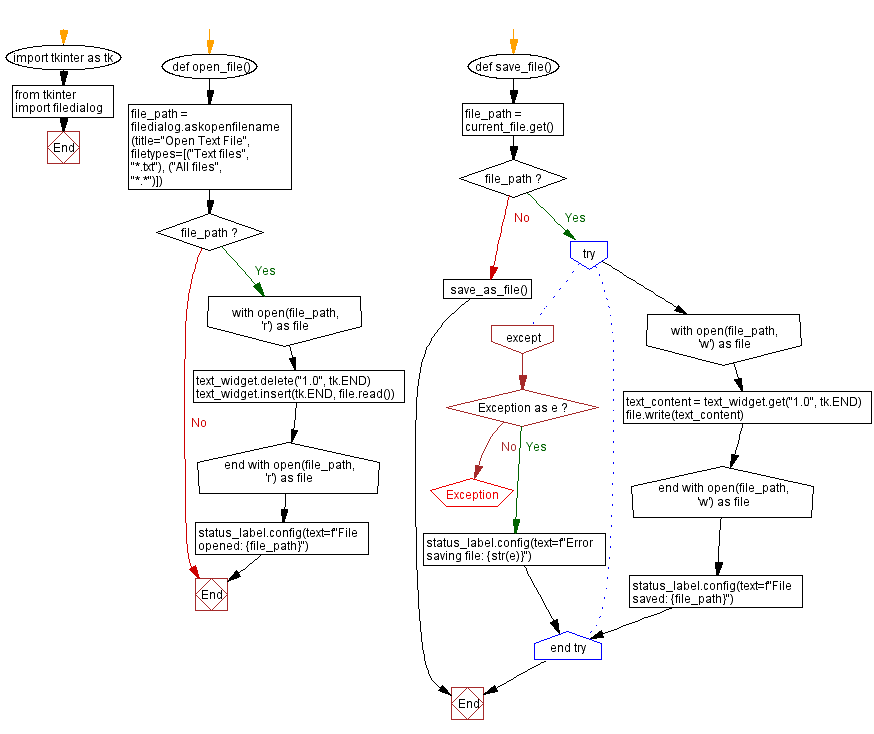
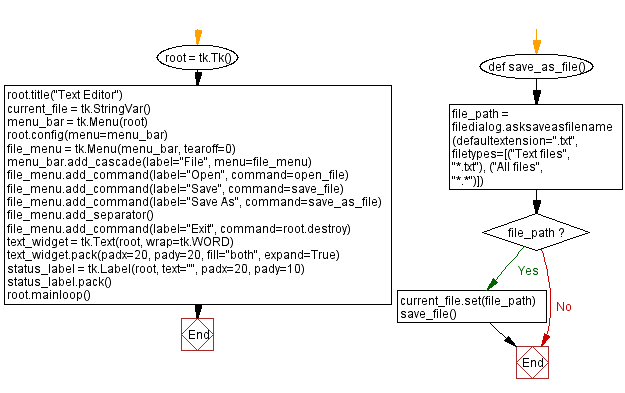
Python Code Editor:
Previous: Save text to file.
Next: Python Tkinter directory file list viewer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics