Creating an image zooming application in Python with Tkinter
Write a Python program that uses the Canvas widget to display an image and allows users to zoom in and out using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from PIL import Image, ImageTk
class ImageZoomApp:
def __init__(self, root):
self.root = root
self.root.title("Image Zoom")
# Create a Canvas widget
self.canvas = tk.Canvas(self.root, bg="white")
self.canvas.pack(fill=tk.BOTH, expand=True)
# Initialize image and image reference
self.image = None
self.tk_image = None
# Load an initial image (you can use your own image file)
self.load_image("image1.png")
# Create Zoom In and Zoom Out buttons
zoom_in_button = tk.Button(self.root, text="Zoom In", command=self.zoom_in)
zoom_out_button = tk.Button(self.root, text="Zoom Out", command=self.zoom_out)
zoom_in_button.pack(side=tk.LEFT)
zoom_out_button.pack(side=tk.LEFT)
# Bind mouse wheel events for zooming
self.canvas.bind("<Button-4>", self.zoom_in)
self.canvas.bind("<Button-5>", self.zoom_out)
def load_image(self, filename):
# Load the image using PIL (Python Imaging Library)
self.image = Image.open(filename)
self.tk_image = ImageTk.PhotoImage(self.image)
# Display the image on the Canvas
self.canvas.create_image(0, 0, anchor=tk.NW, image=self.tk_image)
def zoom_in(self, event=None):
# Increase the image size by a factor (e.g., 1.2)
self.image = self.image.resize((int(self.image.width * 1.2), int(self.image.height * 1.2)), Image.ANTIALIAS)
self.tk_image = ImageTk.PhotoImage(self.image)
self.canvas.delete("all")
self.canvas.create_image(0, 0, anchor=tk.NW, image=self.tk_image)
def zoom_out(self, event=None):
# Decrease the image size by a factor (e.g., 0.8)
self.image = self.image.resize((int(self.image.width * 0.8), int(self.image.height * 0.8)), Image.ANTIALIAS)
self.tk_image = ImageTk.PhotoImage(self.image)
self.canvas.delete("all")
self.canvas.create_image(0, 0, anchor=tk.NW, image=self.tk_image)
if __name__ == "__main__":
root = tk.Tk()
app = ImageZoomApp(root)
root.mainloop()
Explanation:
In the exercise above -
Import the necessary libraries:
- 'tkinter': Provides functions for creating the GUI.
- 'PIL' (Python Imaging Library): Used for opening, resizing, and displaying images.
Define a class 'ImageZoomApp' to encapsulate the image zooming application functionality.
In the '__init__' method:
- Initialize the main application window ('root') with the title "Image Zoom."
- Create a Canvas widget ('self.canvas') to display the image.
- Initialize image-related attributes ('self.image' and 'self.tk_image') to 'None'.
- Load an initial image using the 'load_image' method (replace '"image1.png"' with the path to your own image).
- Create "Zoom In" and "Zoom Out" buttons using the 'tk.Button' widget and associate them with the respective methods ('self.zoom_in' and 'self.zoom_out').
- Bind mouse wheel events to the Canvas for zooming in and out using '<Button-4>' (scroll up) and '<Button-5>' (scroll down).
Define the 'load_image' method:
- This method loads an image from the specified file using PIL.
- Converts the loaded image into a Tkinter PhotoImage ('self.tk_image') to display it on the Canvas.
- Displays the image on the Canvas using 'self.canvas.create_image'.
Define the 'zoom_in' method:
- Increases the image size by a factor of 1.2 (zooming in) using the 'resize' method from PIL.
- Updates 'self.image' and 'self.tk_image' with the resized image and PhotoImage.
- Clears the Canvas ('self.canvas.delete("all"') and redraws the zoomed-in image.
Define the 'zoom_out' method:
- Decreases the image size by a factor of 0.8 (zooming out) using the 'resize' method from PIL.
- Updates 'self.image' and 'self.tk_image' with the resized image and PhotoImage.
- Clears the Canvas ('self.canvas.delete("all"') and redraws the zoomed-out image.
In the main section:
- Create a Tkinter main window ('root').
- Create an instance of the 'ImageZoomApp' class ('app') to start the application.
- Start the Tkinter main event loop with 'root.mainloop()'.
You will see an initial image when running this program. To zoom in and out of the image, click the "Zoom In" and "Zoom Out" buttons or use the mouse wheel.
Output:
Flowchart:
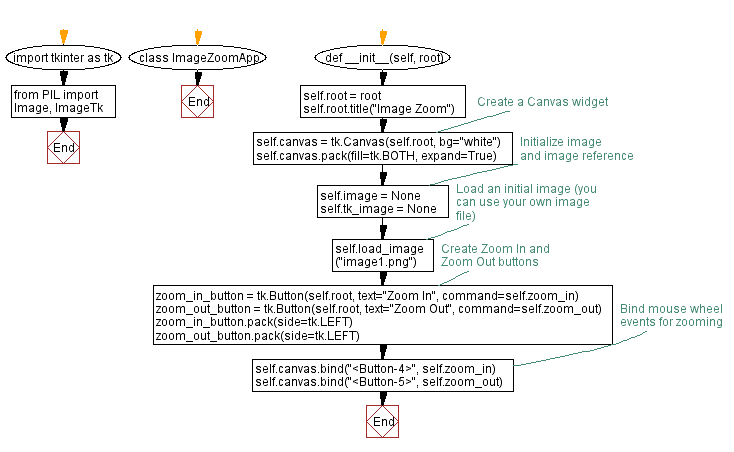

Python Code Editor:
Previous: Creating a colorful canvas drawing program with Python and Tkinter.
Next: Create a 'Catch the Ball' game with Python and Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics