Building a whiteboard application with Python and Tkinter
Write a Python program that implements a Tkinter-based whiteboard application where users can draw, erase, and clear the canvas.
Sample Solution:
Python Code:
import tkinter as tk
class WhiteboardApp:
def __init__(self, root):
self.root = root
self.root.title("Whiteboard")
self.canvas = tk.Canvas(root, width=300, height=300, bg="white")
self.canvas.pack()
self.draw_button = tk.Button(root, text="Draw", command=self.start_draw)
self.draw_button.pack(side=tk.LEFT)
self.erase_button = tk.Button(root, text="Erase", command=self.start_erase)
self.erase_button.pack(side=tk.LEFT)
self.clear_button = tk.Button(root, text="Clear", command=self.clear_canvas)
self.clear_button.pack(side=tk.LEFT)
self.drawing = False
self.erasing = False
self.last_x, self.last_y = None, None
self.canvas.bind("<Button-1>", self.start_action)
self.canvas.bind("<B1-Motion>", self.draw_or_erase)
self.canvas.bind("<ButtonRelease-1>", self.stop_action)
def start_action(self, event):
if self.drawing:
self.start_draw(event)
elif self.erasing:
self.start_erase(event)
def stop_action(self, event):
if self.drawing:
self.stop_draw(event)
elif self.erasing:
self.stop_erase(event)
def start_draw(self, event=None):
self.drawing = True
self.erasing = False
if event:
self.last_x, self.last_y = event.x, event.y
def stop_draw(self, event=None):
self.drawing = False
self.erasing = False
def start_erase(self, event=None):
self.erasing = True
self.drawing = False
if event:
self.last_x, self.last_y = event.x, event.y
def stop_erase(self, event=None):
self.erasing = False
self.drawing = False
def draw_or_erase(self, event):
if self.drawing:
x, y = event.x, event.y
self.canvas.create_line(self.last_x, self.last_y, x, y, fill="black", width=2)
self.last_x, self.last_y = x, y
elif self.erasing:
x, y = event.x, event.y
self.canvas.create_rectangle(x - 5, y - 5, x + 5, y + 5, fill="white", outline="white")
def clear_canvas(self):
self.canvas.delete("all")
if __name__ == "__main__":
root = tk.Tk()
app = WhiteboardApp(root)
root.mainloop()
Explanation:
In the exercise above -
- Import the Tkinter library (tkinter).
- Create a WhiteboardApp class to manage the whiteboard application.
- Users can switch between drawing and erasing modes by clicking the "Draw" and "Erase" buttons.
- The clear_canvas method clears the entire canvas.
- Mouse events track drawing and erasing actions.
- The canvas is set up to capture mouse button events and motion events.
- The main event loop, root.mainloop(), starts the Tkinter application.
Output:
Flowchart:
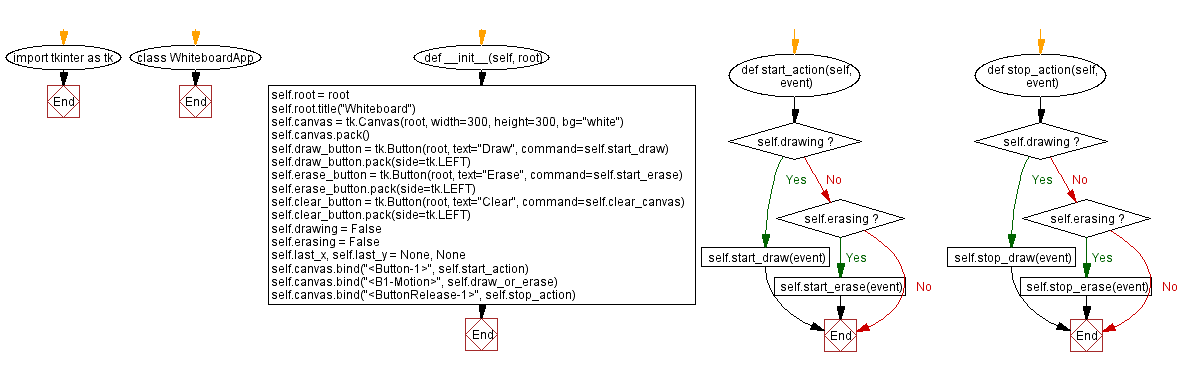
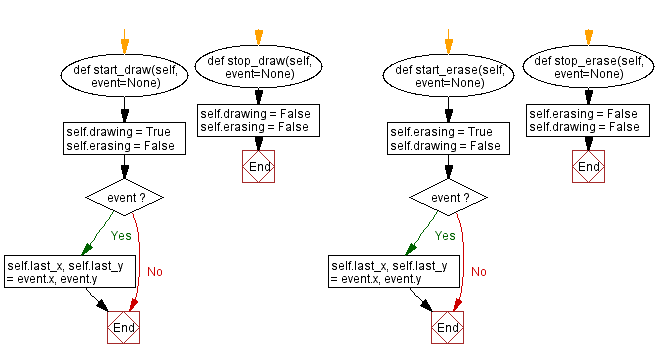
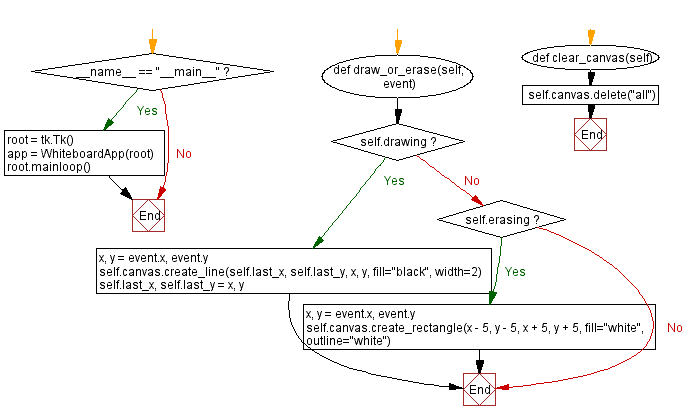
Python Code Editor:
Previous: Creating a line graph with Python and Tkinter's canvas.
Next: Creating a bouncing ball animation with Python and Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics