Building a paint application with Python and Tkinter
Write a Python program to design a paint application using Tkinter where the user can draw on the Canvas widget with different colors.
Sample Solution:
Python Code:
import tkinter as tk
# Global variables to track drawing state
drawing = False
last_x, last_y = None, None
pen_color = "black"
def start_drawing(event):
global drawing
drawing = True
global last_x, last_y
last_x, last_y = event.x, event.y
def stop_drawing(event):
global drawing
drawing = False
def draw(event):
global last_x, last_y
if drawing:
x, y = event.x, event.y
canvas.create_line((last_x, last_y, x, y), fill=pen_color, width=2)
last_x, last_y = x, y
def change_color(new_color):
global pen_color
pen_color = new_color
root = tk.Tk()
root.title("Paint Application")
canvas = tk.Canvas(root, width=400, height=300, bg="white")
canvas.pack()
colors = ["red", "green", "blue", "black", "orange", "pink"]
color_buttons = []
for color in colors:
color_buttons.append(tk.Button(root, text=color.capitalize(), bg=color, command=lambda c=color: change_color(c)))
color_buttons[-1].pack(side=tk.LEFT)
canvas.bind("<Button-1>", start_drawing)
canvas.bind("<ButtonRelease-1>", stop_drawing)
canvas.bind("<B1-Motion>", draw)
root.mainloop()
Explanation:
In the exercise above -
- Import the Tkinter library (tkinter).
- Define global variables to track drawing state (drawing), last position ('last_x', 'last_y'), and pen color ('pen_color').
- Define functions to handle drawing: start_drawing(event): Sets drawing to True and records the initial mouse position. stop_drawing(event): Sets drawing to False when the mouse button is released.
- draw(event): Draws lines on the canvas if drawing is True, connecting the last recorded position to the current mouse position.
- Create the main "Tkinter" window (root) with the title "Paint Application."
- Create a canvas (canvas) for drawing with a white background.
- Define a list of color names (colors) for the pen.
- Create color buttons for each color in the list, associating each button with the change_color() function to change the pen color.
- Bind mouse events to the canvas:
- The main event loop, root.mainloop(), starts the Tkinter application.
Output:
Flowchart:
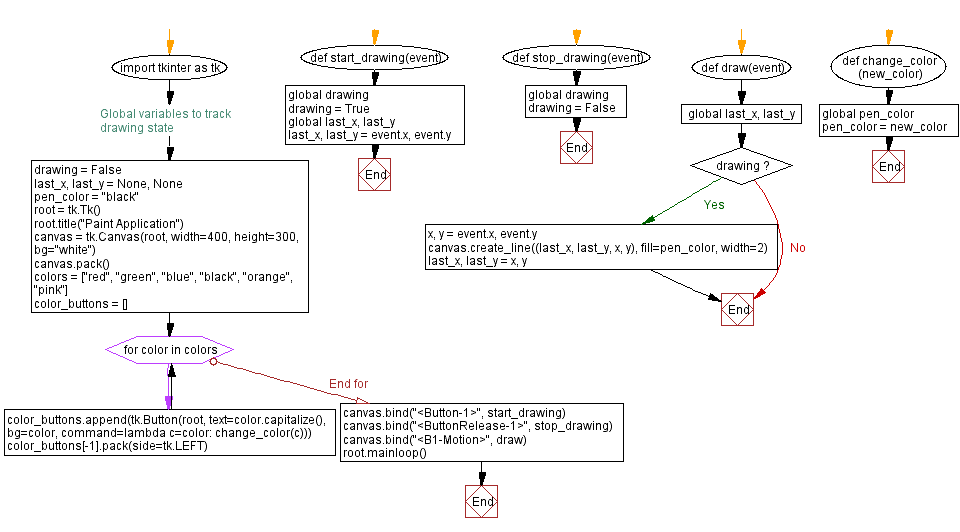
Go to:
Previous: Creating a Blue rectangle on a Tkinter canvas widget.
Next: Building a drawing program with Python and Tkinter.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.