Python Tkinter canvas shape editor: Drawing and manipulating shapes
Write a Python program that allows the user to draw and manipulate geometric shapes (e.g., rectangle, circle) on the "Canvas" using Tkinter.
Sample Solution:
Python Code:
Explanation:
In the exercise above -
- Import the Tkinter library (tkinter).
- Define the ShapeEditorApp class:
- The constructor init initializes the application and creates the main window (root).
- The main window's title is set to "Shape Editor."
- Create a Canvas widget:
- A "Canvas" widget is created within the main window to serve as the drawing area. It's configured with a white background and expand to fill the available space.
- Initialize shape-related variables:
- current_shape stores the currently selected shape ("rectangle" or "circle").
- start_x and start_y store the starting coordinates when drawing shapes.
- current_shape_item stores the currently drawn shape as a Canvas item.
- Create buttons:
- Three buttons are created: "Rectangle," "Circle," and "Clear."
- The buttons are associated with functions (create_rectangle, create_circle, and clear_canvas) that will be called when the buttons are clicked.
- The buttons are packed (placed) on the main window in a horizontal layout using the pack method.
- Bind mouse events:
- Three mouse events are bound to the Canvas widget:
- <Button-1> is bound to the start_draw method when the left mouse button is clicked.
- <B1-Motion> is bound to the draw_shape method when the left mouse button is moved.
- <ButtonRelease-1> is bound to the stop_draw method when the left mouse button is released.
- Define functions for button actions:
- create_rectangle and create_circle set the current_shape variable to "rectangle" or "circle" when the respective buttons are clicked.
- start_draw initializes the drawing process by storing the starting coordinates and creating an initial shape (either a rectangle or a circle) at the starting point.
- draw_shape updates the shape's coordinates as the mouse is moved, allowing the user to draw the shape.
- stop_draw resets the current_shape_item to None when the drawing process is complete.
- clear_canvas deletes all items on the "Canvas" to clear the drawing.
- Create the main application window:
- The root window is created using tk.Tk().
- An instance of the "ShapeEditorApp" class is created, which initializes the GUI and sets up the event handlers.
- Start the Tkinter main loop.
Output:
Flowchart:
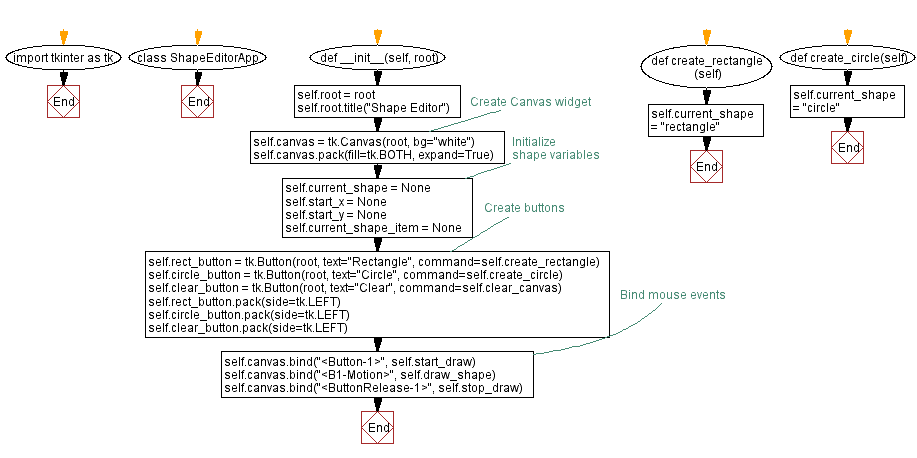
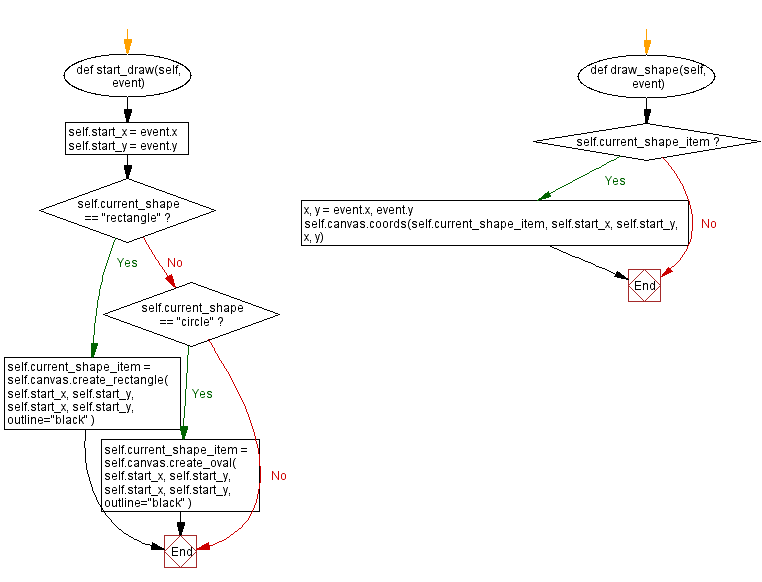
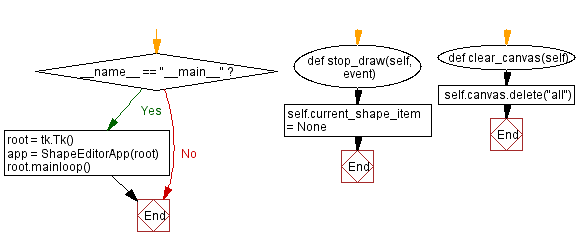
Go to:
Previous: Build interactive charts and graphs with Python and Tkinter.
Next: Create an interactive geometric shape editor with Python and Tkinter.
Python Code Editor:
What is the difficulty level of this exercise?
Based on 68 votes, average difficulty level of this exercise is Medium
.
Test your Programming skills with w3resource's quiz.