Create a Python Tkinter application with color picker
Python tkinter Basic: Exercise-19 with Solution
Write a Python program that creates a Tkinter application that allows users to select and display their favorite color using a color picker.
Sample Solution:
Python Code:
import tkinter as tk
from tkcolorpicker import askcolor
# Function to open the color picker and display the selected color
def choose_color():
color = askcolor()[1] # askcolor() returns (color, color_name)
if color:
color_label.config(text=f"Selected Color: {color}", bg=color)
# Create the main window
parent = tk.Tk()
parent.title("Color Picker")
# Create a label to display the selected color
color_label = tk.Label(parent, text="Selected Color: None", font=("Helvetica", 14), padx=10, pady=10)
color_label.pack()
# Create a button to open the color picker
choose_button = tk.Button(parent, text="Choose Color", command=choose_color)
choose_button.pack(pady=10)
# Start the Tkinter event loop
parent.mainloop()
Explanation:
In the exercise above -
- First we import the "tkinter" library and the askcolor function from "tkcolorpicker".
- The "choose_color()" function is defined to open the color picker dialog using askcolor(), which returns the selected color. We then update the label's text and background color to display the selected color.
- The main window is created using tk.Tk().
- A label is created to display the selected color, and a button is created to open the color picker dialog.
- The program starts the Tkinter event loop using root.mainloop().
Sample Output:
Flowchart:
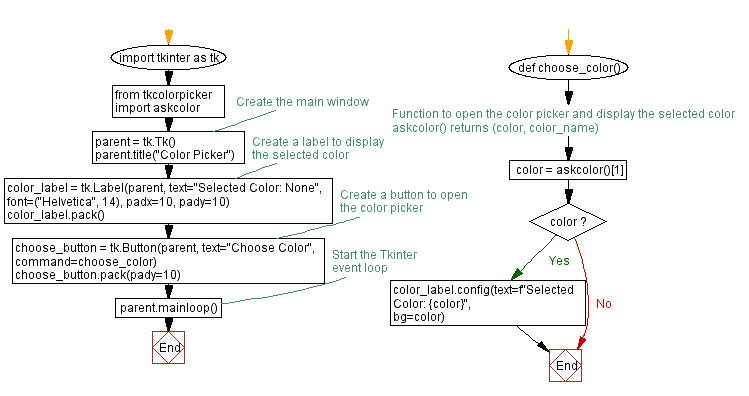
Python Code Editor:
Previous: Create a Python GUI program to close a window.
Next: Create a Python Tkinter timer application.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/tkinter/python-tkinter-basic-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics