Create a Python GUI program to close a window
Write a Python GUI program to create a window that closes when a "Close" button is clicked.
Sample Solution:
Python Code:
import tkinter as tk
# Function to close the window
def close_window():
parent.destroy()
# Create the main window
parent = tk.Tk()
parent.title("Close Window Example")
# Create a label
label = tk.Label(parent, text="Click the 'Close' button to close this window.")
label.pack(padx=25, pady=25)
# Create a close button
close_button = tk.Button(parent, text="Close", command=close_window)
close_button.pack()
# Start the Tkinter event loop
parent.mainloop()
Explanation:
In the exercise above -
- First we import the 'tkinter' library and create the main window using tk.Tk().
- Next we define a function called "close_window()" that calls root.destroy() to close the main window.
- A label and a "Close" button are added to the window. The button's command parameter is set to 'close_window', so clicking the button will execute the "close_window()" function.
- The program starts the Tkinter event loop using parent.mainloop(), which keeps the GUI window running.
Sample Output:
Flowchart:
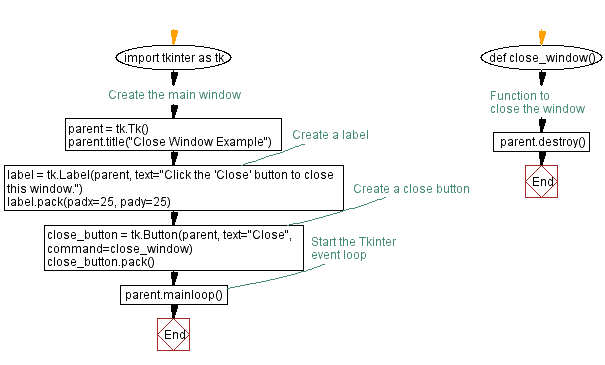
Go to:
Previous: Create interactive tooltips in a Python Tkinter window.
Next: Create a Python Tkinter application with color picker.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.