Create a login form in Python with Tkinter
Write a Python program to create a Tkinter-based login form with input fields for userid and password.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import messagebox
# Function to validate the login
def validate_login():
userid = username_entry.get()
password = password_entry.get()
# You can add your own validation logic here
if userid == "admin" and password == "password":
messagebox.showinfo("Login Successful", "Welcome, Admin!")
else:
messagebox.showerror("Login Failed", "Invalid username or password")
# Create the main window
parent = tk.Tk()
parent.title("Login Form")
# Create and place the username label and entry
username_label = tk.Label(parent, text="Userid:")
username_label.pack()
username_entry = tk.Entry(parent)
username_entry.pack()
# Create and place the password label and entry
password_label = tk.Label(parent, text="Password:")
password_label.pack()
password_entry = tk.Entry(parent, show="*") # Show asterisks for password
password_entry.pack()
# Create and place the login button
login_button = tk.Button(parent, text="Login", command=validate_login)
login_button.pack()
# Start the Tkinter event loop
parent.mainloop()
Explanation:
In the exercise above -
- First we import the necessary modules from Tkinter.
- The "validate_login()" function validates login credentials.
- Next we create the main window with the title "Login Form."
- Input fields for userid and password are created using tk.Entry widgets. The show="*" option for the password entry field displays asterisks.
- A login button is created using tk.Button, and its command is set to the validate_login function.
- The Tkinter event loop (root.mainloop()) keeps the GUI running and responsive to user interactions.
Sample Output:
Flowchart:
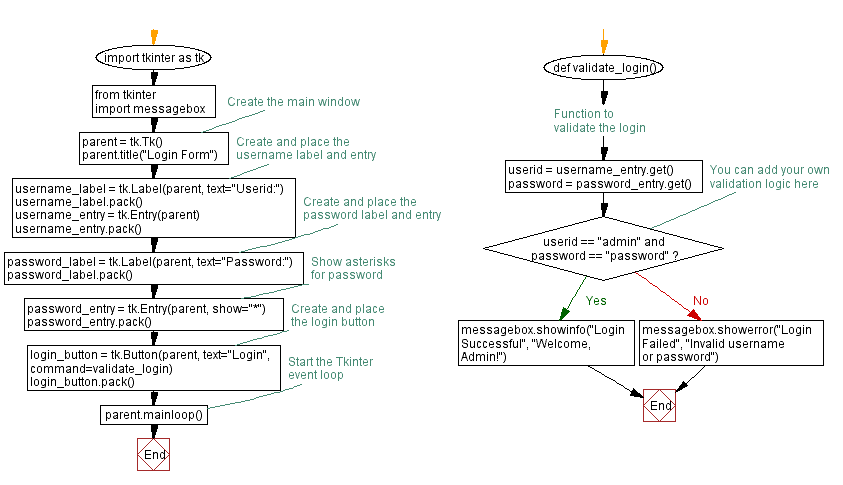
Python Code Editor:
Previous: Create a temperature converter in Python using Tkinter.
Next: Create interactive tooltips in a Python Tkinter window.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics