Create a digital clock in Python using Tkinter
Python tkinter Basic: Exercise-14 with Solution
Write a Python program to implement a Tkinter-based digital clock that displays the current time on a label.
Sample Solution:
Python Code:
import tkinter as tk
import time
# Function to update the label text with the current time
def update_time():
current_time = time.strftime('%H:%M:%S')
clock_label.config(text=current_time)
root.after(1000, update_time) # Update every 1000 milliseconds (1 second)
# Create the main window
root = tk.Tk()
root.title("Digital Clock")
# Create a label to display the time
clock_label = tk.Label(root, text="", font=("Helvetica", 48))
clock_label.pack(padx=20, pady=20)
# Start updating the time
update_time()
# Start the Tkinter event loop
root.mainloop()
Explanation:
In the exercise above -
- Import the tkinter library as tk and the time module.
- The "update_time()" function updates the label's text with the current time. We use time.strftime('%H:%M:%S') to get the current time in the format "HH:MM:SS." The label's text is updated every 1000 milliseconds (1 second) using the root.after method.
- Create the main window with the title "Digital Clock."
- A label (clock_label) is created to display the time. We set the font size to 48 points for a larger display.
- Update the time by calling the "update_time()" function.
- With root.mainloop(), we start the Tkinter event loop, which keeps the GUI running and updates the time.
Sample Output:
Flowchart:
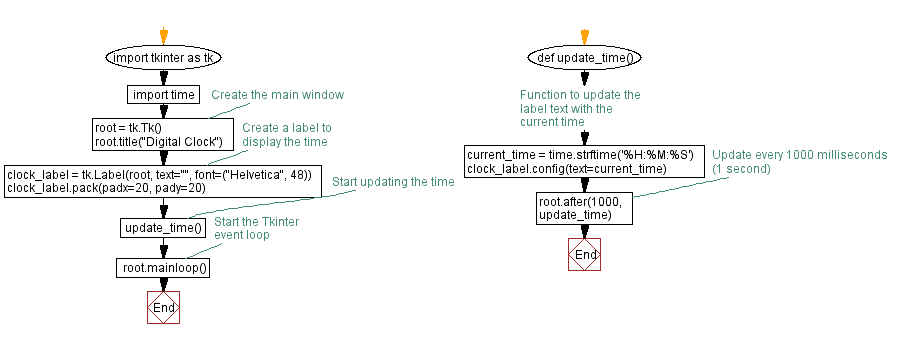
Python Code Editor:
Previous: Build a simple calculator in Python using Tkinter.
Next: Create a temperature converter in Python using Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics