Add an image to a Tkinter window in Python
Write a Python GUI program to add an image (e.g., a logo) to a Tkinter window.
Sample Solution:
Python Code:
import tkinter as tk
from PIL import Image, ImageTk
# Create the main window
parent = tk.Tk()
parent.title("Image in Tkinter")
# Load and display an image
#(replace 'your_logo.png' with the path to your image file)
image = Image.open('w3r_logo.png')
image = ImageTk.PhotoImage(image)
# Create a label to display the image
image_label = tk.Label(parent, image=image)
image_label.pack()
# Start the Tkinter event loop
parent.mainloop()
In the exercise above,
- Load an image using the PIL library and open it with Image.open().
- Create an ImageTk.PhotoImage object from the loaded image.
- Create a label widget (tk.Label) and set its image to the ImageTk.PhotoImage object.
- Finally, use pack() to display the label with the image in the "Tkinter" window.
The "Tkinter" window will display the specified image (e.g., your logo) when we run this program.
Sample Output:
Flowchart:
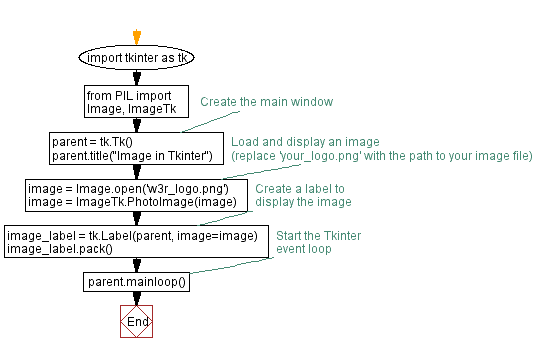
Python Code Editor:
Previous: Create a Python GUI window with custom background color.
Next: Build a simple calculator in Python using Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics