Customize labels and buttons in Python Tkinter
Write a Python program that customizes the appearance of labels and buttons (e.g., fonts, colors) using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
# Create the main window
parent = tk.Tk()
parent.title("Customizing Labels and Buttons")
# Customize label appearance
label = tk.Label(parent, text="Custom Label", font=("Arial", 18), fg="white", bg="red")
label.pack(pady=10)
# Customize button appearance
button = tk.Button(parent, text="Custom Button", font=("Helvetica", 14), fg="white", bg="blue")
button.pack(pady=10)
# Start the Tkinter event loop
parent.mainloop()
In the exercise above,
- Create a label with the text "Custom Label" and customize its appearance by setting the font to Arial with a size of 18, the text color (foreground) to 'white', and the background color to 'red'.
- Create a button with the text "Custom Button" and customize its appearance by setting the font to Helvetica with a size of 14, the text color (foreground) to 'white', and the background color to 'blue'.
Note: Adjust the font family, size, and colors to match your desired appearance. The fg attribute is used to set the foreground (text) color, and the bg attribute is used to set the background color.
Sample Output:
Flowchart:
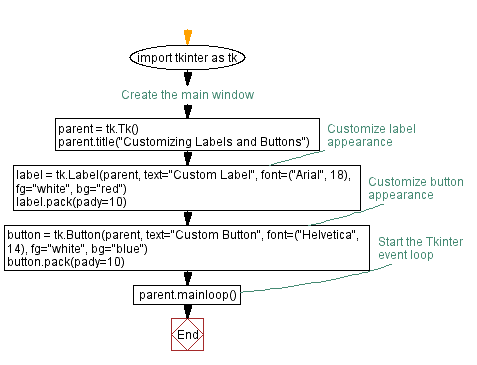
Python Code Editor:
Previous: Python Tkinter Messagebox: Display messages.
Next: Create a Python GUI window with custom background color.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.