Python: Convert a given heterogeneous list of scalars into a string
Convert heterogeneous list to string.
Write a Python program to convert a given heterogeneous list of scalars into a string.
Sample Solution:
Python Code:
# Define a function to convert a heterogeneous list of scalars into a string
def heterogeneous_list_to_str(lst):
# Use the join() method to concatenate the string representations of each element in the list
result = ','.join(str(x) for x in lst)
# Return the resulting string
return result
# Initialize a heterogeneous list of scalars
h_data = ["Red", 100, -50, "green", "w,3,r", 12.12, False]
# Print the original list
print("Original list:")
print(h_data)
# Print a newline for better formatting
print("\nConvert the heterogeneous list of scalars into a string:")
# Call the function to convert the list into a string and print the result
print(heterogeneous_list_to_str(h_data))
Sample Output:
Original list: ['Red', 100, -50, 'green', 'w,3,r', 12.12, False] Convert the heterogeneous list of scalars into a string: Red,100,-50,green,w,3,r,12.12,False
Flowchart:
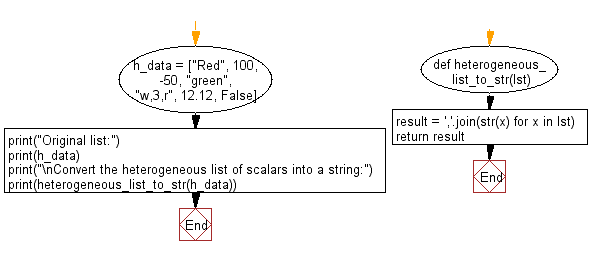
Python Code Editor:
Previous: Write a Python program to remove duplicate words from a given string.
Next: Write a Python program to find the string similarity between two given strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics