Python: Convert a given Bytearray to Hexadecimal string
Bytearray to hexadecimal string.
Write a Python program to convert a given Bytearray to a Hexadecimal string.
Sample Solution:-
Python Code:
Sample Output:
Original Bytearray : [111, 12, 45, 67, 109] Hexadecimal string: 6f0c2d436d
Flowchart:
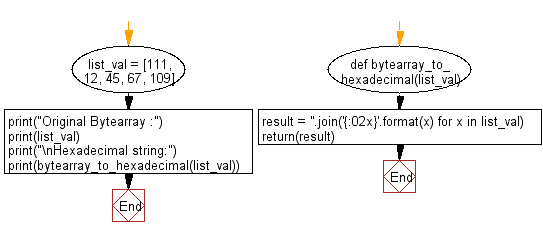
For more Practice: Solve these Related Problems:
- Write a Python program to convert a bytearray into its corresponding hexadecimal string representation using hex().
- Write a Python program to iterate through a bytearray and format each byte as a two-digit hexadecimal number.
- Write a Python program to use a loop to convert a given bytearray into a concatenated hexadecimal string.
- Write a Python program to implement a function that takes a bytearray and returns a lower-case hexadecimal string without prefixes.
Go to:
Previous: Write a Python program to swap cases of a given string.
Next: Write a Python program to delete all occurrences of a specified character in a given string.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.